Permalink
Please sign in to comment.
Showing
with
95,566 additions
and 149 deletions.
- 0 README.md
- +2 −1 assets/textures/plants.json
- BIN assets/textures/plants.png
- BIN assets/textures/zombie.png
- +7 −0 css/fonts.css
- +7 −2 index.html
- +42 −5 js/Gui.js
- +16 −15 js/Sun.js
- +4 −0 js/cbd/Component.js
- +39 −3 js/cbd/GameObject.js
- +56 −78 js/main.js
- +95,198 −0 js/phaser/phaser.js
- 0 js/{ → phaser}/phaser.min.js
- +34 −0 js/plants/Character.js
- +14 −3 js/plants/Nut.js
- +5 −1 js/plants/Peashooter.js
- +0 −36 js/plants/Plant.js
- +7 −5 js/plants/Sunflower.js
- +79 −0 js/utils/Class.js
- +7 −0 js/utils/LoadPhaser.js
- 0 js/utils/Resource.js
- +49 −0 js/utils/Util.js
0
README.md
No changes.
3
assets/textures/plants.json
BIN
assets/textures/plants.png
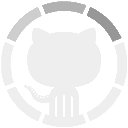
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
BIN
assets/textures/zombie.png
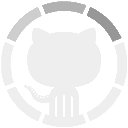
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
7
css/fonts.css
@@ -0,0 +1,7 @@ | ||
+@font-face { | ||
+ font-family: 'VT323'; | ||
+ font-style: normal; | ||
+ font-weight: 400; | ||
+ src: local('../assets/fonts/alterebro-pixel-font.ttf'); | ||
+ unicode-range: U+0000-00FF, U+0131, U+0152-0153, U+02C6, U+02DA, U+02DC, U+2000-206F, U+2074, U+20AC, U+2212, U+2215, U+E0FF, U+EFFD, U+F000; | ||
+} |
9
index.html
47
js/Gui.js
@@ -1,6 +1,43 @@ | ||
-function Gui() { | ||
- | ||
- | ||
- | ||
- | ||
+function Gui(state) { | ||
+ | ||
+ this.heart = game.add.sprite(16, 16, 'plants'); | ||
+ this.heart.width = 64; | ||
+ this.heart.height = 64; | ||
+ this.heart.animations.add('full', ['heart'], 2, true, false); | ||
+ this.heart.animations.add('med', ['heart'], 2, true, false); | ||
+ this.heart.animations.add('low', ['heart'], 2, true, false); | ||
+ this.heart.animations.play('full', 2, true); | ||
+ | ||
+ this.sun = game.add.sprite(80, 16, 'plants'); | ||
+ this.sun.width = 64; | ||
+ this.sun.height = 64; | ||
+ this.sun.animations.add('idle', ['sun'], 2, true, false); | ||
+ this.sun.animations.play('idle', 2, true); | ||
+ | ||
+ var self = this; var i = 0; | ||
+ ['peashooter', 'sunflower', 'nut'].map(function(plant){ | ||
+ | ||
+ var sprite = game.add.sprite(64 * i + 128, 16, 'plants'); | ||
+ | ||
+ sprite.width = 64; | ||
+ sprite.height = 64; | ||
+ sprite.inputEnabled = true; | ||
+ | ||
+ sprite.animations.add('unselected', [plant + '_0'], 2, true, false); | ||
+ sprite.animations.add('selected', [plant + '_1'], 2, true, false); | ||
+ sprite.animations.play('unselected', 2, true); | ||
+ | ||
+ sprite.events.onInputDown.add(function(){ | ||
+ sprite.animations.play('selected', 2, true); | ||
+ }, state); | ||
+ | ||
+ self[plant] = sprite; | ||
+ i++; | ||
+ }); | ||
+ | ||
+ this.sunText = game.add.text(96, 128, '#FontFixBecauseExternalFontsDontWorkWellWithPhaser', { | ||
+ 'font': '40px Arial', | ||
+ 'fillStyle': 'black' | ||
+ }); | ||
+ | ||
} |
31
js/Sun.js
4
js/cbd/Component.js
@@ -1,3 +1,7 @@ | ||
function Component (type) { | ||
this.type = type; | ||
+} | ||
+ | ||
+Component.prototype.update = function () { | ||
+ | ||
} |
42
js/cbd/GameObject.js
134
js/main.js
95,198
js/phaser/phaser.js
95,198 additions,
0 deletions
not shown because the diff is too large. Please use a local Git client to view these changes.
0
js/phaser.min.js → js/phaser/phaser.min.js
File renamed without changes.
34
js/plants/Character.js
@@ -0,0 +1,34 @@ | ||
+function Character(manager, column, row, sprite_x, sprite_y) { | ||
+ this.type = 'character'; | ||
+ this.manager = manager; | ||
+ | ||
+ this.props = new Props({ | ||
+ column: column, | ||
+ row: row, | ||
+ cooldown: 1000, | ||
+ damage: 0, | ||
+ speed: 100, | ||
+ health: 2 | ||
+ }); | ||
+ | ||
+ this.sprite = game.add.sprite(sprite_x, sprite_y, 'plants'); | ||
+ this.sprite.smoothed = false; | ||
+ this.sprite.width = 64; | ||
+ this.sprite.height = 64; | ||
+ | ||
+ //Common functions | ||
+ | ||
+ this.hit = function(damage){ | ||
+ // Decrease health by the amount of damage, but never allow health to drop below zero | ||
+ this.props.health = Math.max(this.props.health - damage, 0); | ||
+ | ||
+ // If health is zero, mark the plant as dead | ||
+ if (this.props.health <= 0) { | ||
+ this.props.die = true; | ||
+ } | ||
+ }; | ||
+ | ||
+ this.init(); | ||
+} | ||
+ | ||
+Character.__proto__ = Component('character'); |
17
js/plants/Nut.js
@@ -1,12 +1,23 @@ | ||
+function Nut() { | ||
+ Character.apply(this, arguments); | ||
+} | ||
+ | ||
Nut.prototype.init = function (){ | ||
this.last_action_time = 0; | ||
this.props.health = 14; | ||
+ | ||
+ this.sprite.animations.add('idle_1', ['nut_0'], 2, true, false); | ||
+ this.sprite.animations.add('idle_2', ['nut_1'], 2, true, false); | ||
+ this.sprite.animations.add('idle_3', ['nut_2'], 2, true, false); | ||
+ this.sprite.animations.add('idle_4', ['nut_3'], 2, true, false); | ||
+ this.sprite.animations.add('idle_5', ['nut_4'], 2, true, false); | ||
+ this.sprite.animations.add('idle_6', ['nut_5'], 2, true, false); | ||
+ this.sprite.animations.add('idle_7', ['nut_6'], 2, true, false); | ||
+ this.sprite.animations.play('idle_0', 2, true); | ||
}; | ||
Nut.prototype.update = function() { | ||
- | ||
// Update sprite based on health remaining | ||
var idleAnimationIndex = (8 - (this.props.health / 2)); // maps health in range of 1-7 (1 means full health, 7 means almost no health) | ||
- this.sprite.animations.play('idle' + idleAnimationIndex.toString(), 1, false); | ||
- | ||
+ this.sprite.animations.play('idle_' + idleAnimationIndex.toString(), 1, false); | ||
}; |
6
js/plants/Peashooter.js
36
js/plants/Plant.js
@@ -1,36 +0,0 @@ | ||
-function Character(manager, column, row, sprite, sprite_x, sprite_y) { | ||
- | ||
- this.manager = manager; | ||
- | ||
- this.props = new Props({ | ||
- column: column, | ||
- row: row, | ||
- cooldown: 1000, | ||
- damage: 0, | ||
- speed: 100, | ||
- health: 2 | ||
- }); | ||
- | ||
- this.sprite = game.add.sprite(sprite_x, sprite_y, 'plants'); | ||
- this.sprite.smoothed = false; | ||
- this.sprite.width = 64; | ||
- this.sprite.height = 64; | ||
-} | ||
- | ||
-//Common functions | ||
- | ||
-Character.prototype.hit = function (damage) { | ||
- // Decrease health by the amount of damage, but never allow health to drop below zero | ||
- this.props.health = Math.max(this.props.health - damage, 0); | ||
- | ||
- // If health is zero, mark the plant as dead | ||
- if (this.props.health === 0) { | ||
- this.props.die = true; | ||
- } | ||
-} | ||
- | ||
-//PlantTypes | ||
- | ||
-Sunflower = Character; | ||
-Peashooter = Character; | ||
-Nut = Character; |
12
js/plants/Sunflower.js
79
js/utils/Class.js
@@ -0,0 +1,79 @@ | ||
+var PvZ = PvZ || {}; | ||
+ | ||
+ | ||
+// *** Example of creating a simple class within the PvZ namespace ! (PS: we should have namespaces Plants Vs Zombies === PvZ) | ||
+ | ||
+// var Game = PvZ.Class.create('PvZ.Game', function Game(options, dependencies) { | ||
+ | ||
+// this.dependencies = dependencies; | ||
+// this.options = PvZ.Utils.extend({}, this.options, options); | ||
+// this.config = PvZ.Config; | ||
+ | ||
+// this.init(); | ||
+// }); | ||
+ | ||
+// *** this is how you extend a random class lets say you want to make a new player class | ||
+ | ||
+// var Player = PvZ.Class.extend(PvZ.Game.Entity, 'PvZ.Game.Entity.Player', PvZ.Game.Entity); <--- assuming you have entities i think you guys call them characters | ||
+ | ||
+// Player.prototype.init = function() { | ||
+// this.controller = new O.Game.Controller; | ||
+// PvZ.Logger.log("Player Created"); | ||
+ | ||
+// this.x = this.canvas.width / 2; | ||
+// this.y = this.canvas.height / 2; | ||
+ | ||
+// this.radius = 70; | ||
+// this.speed = 10; | ||
+// } | ||
+ | ||
+PvZ.Class = (function() { | ||
+ function makeNameSpace(namespace, constructor){ | ||
+ var parts = namespace.split('.'); | ||
+ var parent = window; | ||
+ | ||
+ //loop thorugh namespace parts | ||
+ var part; | ||
+ for (var i = 0; i < parts.length; i++) { | ||
+ part = parts[i]; | ||
+ if (typeof parent[part] === 'undefined') { | ||
+ if(i === parts.length - 1 && typeof constructor === 'function' ){ | ||
+ parent[part] = constructor; | ||
+ }else{ | ||
+ parent[part] = {}; | ||
+ } | ||
+ } | ||
+ parent = parent[part]; | ||
+ }; | ||
+ | ||
+ return parent; | ||
+ } | ||
+ | ||
+ return { | ||
+ create: function(namespace, constructor) { | ||
+ var newClass = makeNameSpace(namespace, constructor); | ||
+ newClass = constructor; | ||
+ newClass.prototype.fullClassName = namespace; | ||
+ newClass.prototype.toString = function() { | ||
+ return namespace; | ||
+ } | ||
+ | ||
+ return newClass; | ||
+ }, | ||
+ extend: function(parent, namespace, constructor) { | ||
+ | ||
+ var F = function() {}; | ||
+ try{ | ||
+ F.prototype = parent.prototype; | ||
+ } catch (e){ | ||
+ throw new Error('Parent class does not exist..'); | ||
+ } | ||
+ | ||
+ constructor.prototype = new F(); | ||
+ constructor.prototype.parent = parent; | ||
+ constructor.prototype.constructor = constructor; | ||
+ return PvZ.Class.create(namespace, constructor); | ||
+ } | ||
+ } | ||
+ | ||
+})(); |
7
js/utils/LoadPhaser.js
@@ -0,0 +1,7 @@ | ||
+var isPhaserMinified = false; | ||
+ | ||
+if (isPhaserMinified) { | ||
+ loadScript("../phaser/phaser.min.js"); | ||
+} else { | ||
+ loadScript("../phaser/phaser.js"); | ||
+} |
0
js/utils/Resource.js
No changes.
49
js/utils/Util.js
@@ -0,0 +1,49 @@ | ||
+function Util(){} | ||
+ | ||
+Util.prototype.deepExtend = function(out) { | ||
+ out = out || {}; | ||
+ for (var i = 1; i < arguments.length; i++) { | ||
+ var obj = arguments[i]; | ||
+ | ||
+ if (!obj) | ||
+ continue; | ||
+ | ||
+ for (var key in obj) { | ||
+ if (obj.hasOwnProperty(key)) { | ||
+ if (typeof obj[key] === 'object') | ||
+ this.extend(out[key], obj[key]); | ||
+ else | ||
+ out[key] = obj[key]; | ||
+ } | ||
+ } | ||
+ } | ||
+ | ||
+ return out; | ||
+}; | ||
+ | ||
+Util.prototype.extend = function(out) { | ||
+ out = out || {}; | ||
+ | ||
+ for (var i = 1; i < arguments.length; i++) { | ||
+ if (!arguments[i]) | ||
+ continue; | ||
+ | ||
+ for (var key in arguments[i]) { | ||
+ if (arguments[i].hasOwnProperty(key)) | ||
+ out[key] = arguments[i][key]; | ||
+ } | ||
+ } | ||
+ | ||
+ return out; | ||
+}; | ||
+ | ||
+Util.prototype.generateUUID = function() { | ||
+ var d = new Date().getTime(); | ||
+ var uuid = 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function (c) { | ||
+ var r = (d + Math.random() * 16) % 16 | 0; | ||
+ d = Math.floor(d / 16); | ||
+ return (c == 'x' ? r : (r & 0x3 | 0x8)).toString(16); | ||
+ }); | ||
+ return uuid; | ||
+}; | ||
+ |
0 comments on commit
bd291ec