Permalink
Please sign in to comment.
Showing
with
1,233 additions
and 94 deletions.
- +1 −0 .gitignore
- +32 −0 docs/docs/10.10-shallow-compare.zh-CN.md
- +117 −0 docs/docs/10.2-form-input-binding-sugar.zh-CN.md
- +11 −0 docs/docs/10.3-class-name-manipulation.zh-CN.md
- +173 −0 docs/docs/12-context.zh-CN.md
- +29 −0 docs/docs/conferences.zh-CN.md
- +27 −17 docs/docs/getting-started.zh-CN.md
- +78 −58 docs/docs/ref-01-top-level-api.zh-CN.md
- +15 −15 docs/docs/ref-02-component-api.zh-CN.md
- +217 −0 docs/docs/ref-03-component-specs.zh-CN.md
- +90 −0 docs/docs/ref-04-tags-and-attributes.zh-CN.md
- +47 −3 docs/docs/ref-05-events.zh-CN.md
- +16 −0 docs/docs/ref-06-dom-differences.zh-CN.md
- +13 −0 docs/docs/ref-07-special-non-dom-attributes.zh-CN.md
- +193 −0 docs/docs/ref-10-glossary.zh-CN.md
- +1 −1 docs/docs/thinking-in-react.zh-CN.md
- +173 −0 docs/docs/videos.zh-CN.md
1
.gitignore
32
docs/docs/10.10-shallow-compare.zh-CN.md
@@ -0,0 +1,32 @@ | ||
+--- | ||
+id: shallow-compare-zh-CN | ||
+title: 浅比较 | ||
+permalink: shallow-compare-zh-CN.html | ||
+prev: perf-zh-CN.html | ||
+next: advanced-performance-zh-CN.html | ||
+--- | ||
+ | ||
+`shallowCompare` 是一个辅助函数 在以ES6类使用React时,完成和 `PureRenderMixin` 相同的功能。 | ||
+ | ||
+如果你的React组件的绘制函数是 “干净的” (换句话说,它在给定的 props 和 state 下绘制相同的结果),你可以使用这个辅助函数以在某些情况下提升性能。 | ||
+ | ||
+例如: | ||
+ | ||
+```js | ||
+var shallowCompare = require('react-addons-shallow-compare'); | ||
+export class SampleComponent extends React.Component { | ||
+ shouldComponentUpdate(nextProps, nextState) { | ||
+ return shallowCompare(this, nextProps, nextState); | ||
+ } | ||
+ | ||
+ render() { | ||
+ return <div className={this.props.className}>foo</div>; | ||
+ } | ||
+} | ||
+``` | ||
+ | ||
+`shallowCompare` 对当前的 `props` 和 `nextProps`对象 执行一个浅的相等检查,同样对于 `state` 和 `nextState`对象。 | ||
+它 通过迭代比较对象的keys 并在 对象的key值不严格相等时返回false 实现此功能. | ||
+ | ||
+`shallowCompare` 返回 `true` 如果对 props 或 state的浅比较失败,因此组件应该更新。 | ||
+`shallowCompare` 返回 `false` 如果对 props 或 state的浅比较都通过了,因此组件不应该更新。 |
117
docs/docs/10.2-form-input-binding-sugar.zh-CN.md
@@ -0,0 +1,117 @@ | ||
+--- | ||
+id: two-way-binding-helpers-zh-CN | ||
+title: 双向绑定辅助 | ||
+permalink: two-way-binding-helpers-zh-CN.html | ||
+prev: animation-zh-CN.html | ||
+next: test-utils-zh-CN.html | ||
+--- | ||
+ | ||
+`ReactLink` 是一个用React表达双向绑定的简单方法。 | ||
+ | ||
+> 注意: | ||
+> | ||
+> 如果你刚学这个框架,注意 `ReactLink` 对大多数应用是不需要的,应该慎重的使用。 | ||
+ | ||
+在React里,数据单向流动: 从拥有者到子级。这是因为数据只单向流动[the Von Neumann model of computing](https://en.wikipedia.org/wiki/Von_Neumann_architecture)。你可以把它想象为 “单向数据绑定”。 | ||
+ | ||
+然而,有很多应用需要你去读某些数据并回流他们到你的程序。例如,当开发forms,你会常常想更新一些React `state` 当你收到用户输入的时候。或者也许你想在JavaScript完成布局并相应一些DOM元素大小的变化。 | ||
+ | ||
+在React里,你可以用监听 "change" 事件来实现它,从你的数据源(通常是DOM)读取并在你的某个组件调用 `setState()` 。明确的"Closing the data flow loop" 致使了更容易理解和维护的程序。更多信息见[our forms documentation](/react/docs/forms.html). | ||
+ | ||
+双向绑定 -- 隐含的强迫DOM里的某些值总是和某些React `state` 同步 -- 简洁并支持大量多样的应用。 我们提供了 `ReactLink`:设置如上描述的通用数据回流模式的语法糖,或者 "linking" 某些数据结构到 React `state`. | ||
+ | ||
+> 注意: | ||
+> | ||
+> `ReactLink` 只是一层对 `onChange`/`setState()` 模式的薄包装。它没有根本性的改变你的React应用里数据如何流动。 | ||
+ | ||
+## ReactLink: 之前和之后 | ||
+ | ||
+这里有一个简单的 不用 `ReactLink` 的 form 例子: | ||
+ | ||
+```javascript | ||
+var NoLink = React.createClass({ | ||
+ getInitialState: function() { | ||
+ return {message: 'Hello!'}; | ||
+ }, | ||
+ handleChange: function(event) { | ||
+ this.setState({message: event.target.value}); | ||
+ }, | ||
+ render: function() { | ||
+ var message = this.state.message; | ||
+ return <input type="text" value={message} onChange={this.handleChange} />; | ||
+ } | ||
+}); | ||
+``` | ||
+ | ||
+这个工作的很好并且数据如何流动很清晰,然而,当有大量的 form fields时,可能会有些冗长。让我们使用 `ReactLink` 来节省我们的输入: | ||
+ | ||
+```javascript{4,9} | ||
+var LinkedStateMixin = require('react-addons-linked-state-mixin'); | ||
+ | ||
+var WithLink = React.createClass({ | ||
+ mixins: [LinkedStateMixin], | ||
+ getInitialState: function() { | ||
+ return {message: 'Hello!'}; | ||
+ }, | ||
+ render: function() { | ||
+ return <input type="text" valueLink={this.linkState('message')} />; | ||
+ } | ||
+}); | ||
+``` | ||
+ | ||
+`LinkedStateMixin` 添加了一个 `linkState()` 方法到你的React组件。`linkState()` 返回一个 `ReactLink` 包含当前React state值的对象和一个改变它的回调函数。 | ||
+ | ||
+`ReactLink` 对象可以作为props在树中上下传递,所以很容易(显示的)在深层次的组件和高层次的state之间 设置双向绑定。 | ||
+ | ||
+注意 checkboxes 有一个关于他们 `value` 属性的特殊行为,这个行为是 如果checkbox被选中 值会在表单提交时被发送。 `value` 不会 checkbox 选中或是不选中时更新。对于checkboxes,你应该用`checkedLink` 代替 `valueLink`: | ||
+``` | ||
+<input type="checkbox" checkedLink={this.linkState('booleanValue')} /> | ||
+``` | ||
+ | ||
+## 引擎盖下 | ||
+ | ||
+There are two sides to `ReactLink`: the place where you create the `ReactLink` instance and the place where you use it. To prove how simple `ReactLink` is, let's rewrite each side separately to be more explicit. | ||
+ | ||
+### ReactLink Without LinkedStateMixin | ||
+ | ||
+```javascript{5-7,9-12} | ||
+var WithoutMixin = React.createClass({ | ||
+ getInitialState: function() { | ||
+ return {message: 'Hello!'}; | ||
+ }, | ||
+ handleChange: function(newValue) { | ||
+ this.setState({message: newValue}); | ||
+ }, | ||
+ render: function() { | ||
+ var valueLink = { | ||
+ value: this.state.message, | ||
+ requestChange: this.handleChange | ||
+ }; | ||
+ return <input type="text" valueLink={valueLink} />; | ||
+ } | ||
+}); | ||
+``` | ||
+ | ||
+As you can see, `ReactLink` objects are very simple objects that just have a `value` and `requestChange` prop. And `LinkedStateMixin` is similarly simple: it just populates those fields with a value from `this.state` and a callback that calls `this.setState()`. | ||
+ | ||
+### ReactLink Without valueLink | ||
+ | ||
+```javascript | ||
+var LinkedStateMixin = require('react-addons-linked-state-mixin'); | ||
+ | ||
+var WithoutLink = React.createClass({ | ||
+ mixins: [LinkedStateMixin], | ||
+ getInitialState: function() { | ||
+ return {message: 'Hello!'}; | ||
+ }, | ||
+ render: function() { | ||
+ var valueLink = this.linkState('message'); | ||
+ var handleChange = function(e) { | ||
+ valueLink.requestChange(e.target.value); | ||
+ }; | ||
+ return <input type="text" value={valueLink.value} onChange={handleChange} />; | ||
+ } | ||
+}); | ||
+``` | ||
+ | ||
+The `valueLink` prop is also quite simple. It simply handles the `onChange` event and calls `this.props.valueLink.requestChange()` and also uses `this.props.valueLink.value` instead of `this.props.value`. That's it! |
11
docs/docs/10.3-class-name-manipulation.zh-CN.md
@@ -0,0 +1,11 @@ | ||
+--- | ||
+id: class-name-manipulation-zh-CN | ||
+title: 类名操纵 | ||
+permalink: class-name-manipulation-zh-CN.html | ||
+prev: two-way-binding-helpers-zh-CN.html | ||
+next: test-utils-zh-CN.html | ||
+--- | ||
+ | ||
+> NOTE: | ||
+> | ||
+> 此模块已被弃用; 用 [JedWatson/classnames](https://github.com/JedWatson/classnames) 替代. |
173
docs/docs/12-context.zh-CN.md
@@ -0,0 +1,173 @@ | ||
+--- | ||
+id: context-zh-CN | ||
+title: Context | ||
+permalink: context-zh-CN.html | ||
+prev: advanced-performance-zh-CN.html | ||
+--- | ||
+ | ||
+React最大的优势之一是他很容易从你的React组件里跟踪数据流动。当你看着一个组件,你可以很容易准确看出哪个props被传入,这让你的APP很容易推断。 | ||
+ | ||
+偶尔,你想通过组件树传递数据,而不在每一级上手工下传prop,React的 "context" 让你做到这点。 | ||
+ | ||
+> 注意: | ||
+> | ||
+> Context是一个先进的实验性特性.这个 API 很可能在未来版本变化. | ||
+> | ||
+> 大多数应用将不会需要用到 context. 尤其是如果你刚开始用React,你很可能不会想用 context.使用 context 将会使你的代码很难理解因为它让数据流不清晰.它类似于在你的应用里使用全局变量传递state. | ||
+> | ||
+> **如果你必须使用 context ,保守的使用它** | ||
+> | ||
+> 不论你正在创建一个应用或者是库,试着分离你对 context 的使用到一个小区域,并尽可能避免直接使用 context API,以便在API变动时容易升级. | ||
+ | ||
+## 从树里自动传递info | ||
+ | ||
+假设你有一个这样的结构: | ||
+ | ||
+```javascript | ||
+var Button = React.createClass({ | ||
+ render: function() { | ||
+ return ( | ||
+ <button style={{'{{'}}background: this.props.color}}> | ||
+ {this.props.children} | ||
+ </button> | ||
+ ); | ||
+ } | ||
+}); | ||
+ | ||
+var Message = React.createClass({ | ||
+ render: function() { | ||
+ return ( | ||
+ <div> | ||
+ {this.props.text} <Button color={this.props.color}>Delete</Button> | ||
+ </div> | ||
+ ); | ||
+ } | ||
+}); | ||
+ | ||
+var MessageList = React.createClass({ | ||
+ render: function() { | ||
+ var color = "purple"; | ||
+ var children = this.props.messages.map(function(message) { | ||
+ return <Message text={message.text} color={color} />; | ||
+ }); | ||
+ return <div>{children}</div>; | ||
+ } | ||
+}); | ||
+``` | ||
+ | ||
+在这里例子里,我们手工穿透一个 `color` prop 以便于恰当格式化 `Button` 和 `Message` 组件.主题是一个很好的例子,当你可能想整个子树都可以访问一部分信息时(比如color). 使用 context 我们可以自动传过这个树: | ||
+ | ||
+```javascript{2-4,7,18,25-30,33} | ||
+var Button = React.createClass({ | ||
+ contextTypes: { | ||
+ color: React.PropTypes.string | ||
+ }, | ||
+ render: function() { | ||
+ return ( | ||
+ <button style={{'{{'}}background: this.context.color}}> | ||
+ {this.props.children} | ||
+ </button> | ||
+ ); | ||
+ } | ||
+}); | ||
+ | ||
+var Message = React.createClass({ | ||
+ render: function() { | ||
+ return ( | ||
+ <div> | ||
+ {this.props.text} <Button>Delete</Button> | ||
+ </div> | ||
+ ); | ||
+ } | ||
+}); | ||
+ | ||
+var MessageList = React.createClass({ | ||
+ childContextTypes: { | ||
+ color: React.PropTypes.string | ||
+ }, | ||
+ getChildContext: function() { | ||
+ return {color: "purple"}; | ||
+ }, | ||
+ render: function() { | ||
+ var children = this.props.messages.map(function(message) { | ||
+ return <Message text={message.text} />; | ||
+ }); | ||
+ return <div>{children}</div>; | ||
+ } | ||
+}); | ||
+``` | ||
+ | ||
+通过添加 `childContextTypes` 和 `getChildContext` 到 `MessageList` ( context 提供),React下传信息到子树中的任何组件(在这个例子中, `Button`可以由定义 `contextTypes`来访问它). | ||
+ | ||
+如果 `contextTypes` 没有定义,那么 `this.context` 将是一个空对象. | ||
+ | ||
+## 父子耦合 | ||
+ | ||
+Context 同样可以使你构建这样的 APT: | ||
+ | ||
+```javascript | ||
+<Menu> | ||
+ <MenuItem>aubergine</MenuItem> | ||
+ <MenuItem>butternut squash</MenuItem> | ||
+ <MenuItem>clementine</MenuItem> | ||
+</Menu> | ||
+``` | ||
+ | ||
+通过在 `Menu` 组件下传相关的信息,每个 `MenuItem` 可以与包含他们的 `Menu` 组件沟通. | ||
+ | ||
+**在你用这个API构建组件以前,考虑一下是否有清晰的替代方案** 我们 喜欢像这样简单的用数组传递items: | ||
+ | ||
+```javascript | ||
+<Menu items={['aubergine', 'butternut squash', 'clementine']} /> | ||
+``` | ||
+ | ||
+记住你同样可以在props里传递整个React组件,如果你想. | ||
+ | ||
+## 在生命周期方法里引用 context | ||
+ | ||
+如果 `contextTypes` 在一个组件中定义,接下来的生命周期方法会收到一个额外的参数, `context` 对象: | ||
+ | ||
+```javascript | ||
+void componentWillReceiveProps( | ||
+ object nextProps, object nextContext | ||
+) | ||
+ | ||
+boolean shouldComponentUpdate( | ||
+ object nextProps, object nextState, object nextContext | ||
+) | ||
+ | ||
+void componentWillUpdate( | ||
+ object nextProps, object nextState, object nextContext | ||
+) | ||
+ | ||
+void componentDidUpdate( | ||
+ object prevProps, object prevState, object prevContext | ||
+) | ||
+``` | ||
+ | ||
+## 在无状态函数组件里引用 context | ||
+ | ||
+无状态函数同样能够引用 `context` 如果 `contextTypes` 被定义为函数的属性.下面的代码展示了被写为无状态函数组件的 `Button` 组件. | ||
+ | ||
+```javascript | ||
+function Button(props, context) { | ||
+ return ( | ||
+ <button style={{'{{'}}background: context.color}}> | ||
+ {props.children} | ||
+ </button> | ||
+ ); | ||
+} | ||
+Button.contextTypes = {color: React.PropTypes.string}; | ||
+``` | ||
+ | ||
+## 什么时候不用 context | ||
+ | ||
+正像全局变量是在写清晰代码时最好要避免的,你应该在大多数情况下避免使用context. 特别是,在用它来"节省输入"和代替显示传入props时要三思. | ||
+ | ||
+context最好的使用场景是隐式的传入登录的用户,当前的语言,或者主题信息.要不然所有这些可能就是全局变量,但是context让你限定他们到一个单独的React树里. | ||
+ | ||
+不要用context在组件里传递你的模型数据.通过树显示的传递你的数据更容易理解.使用context使你的组件更耦合和不可复用,因为 依赖于他们在哪里渲染,他们会表现不同的行为. | ||
+ | ||
+## 已知的限制 | ||
+ | ||
+如果一个由组件提供的context值变动,使用那个值的子级不会更新,如果一个直接的父级从 `shouldComponentUpdate` 返回 `false` .详见 issue [#2517](https://github.com/facebook/react/issues/2517) . |
29
docs/docs/conferences.zh-CN.md
@@ -0,0 +1,29 @@ | ||
+--- | ||
+id: conferences-zh-CN | ||
+title: 会议 | ||
+permalink: conferences-zh-CN.html | ||
+prev: thinking-in-react-zh-CN.html | ||
+next: videos-zh-CN.html | ||
+--- | ||
+ | ||
+### React.js Conf 2015 | ||
+一月 28 & 29 | ||
+ | ||
+[Website](http://conf.reactjs.com/) - [Schedule](http://conf.reactjs.com/schedule.html) - [Videos](https://www.youtube-nocookie.com/playlist?list=PLb0IAmt7-GS1cbw4qonlQztYV1TAW0sCr) | ||
+ | ||
+<iframe width="650" height="315" src="//www.youtube-nocookie.com/embed/KVZ-P-ZI6W4?list=PLb0IAmt7-GS1cbw4qonlQztYV1TAW0sCr" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+### ReactEurope 2015 | ||
+七月 2 & 3 | ||
+ | ||
+[Website](http://www.react-europe.org/) - [Schedule](http://www.react-europe.org/#schedule) | ||
+ | ||
+### Reactive 2015 | ||
+十一月 2-4 | ||
+ | ||
+[Website](https://reactive2015.com/) - [Schedule](https://reactive2015.com/schedule_speakers.html#schedule) | ||
+ | ||
+### ReactEurope 2016 | ||
+六月 2 & 3 | ||
+ | ||
+[Website](http://www.react-europe.org/) - [Schedule](http://www.react-europe.org/#schedule) |
44
docs/docs/getting-started.zh-CN.md
136
docs/docs/ref-01-top-level-api.zh-CN.md
30
docs/docs/ref-02-component-api.zh-CN.md
217
docs/docs/ref-03-component-specs.zh-CN.md
@@ -0,0 +1,217 @@ | ||
+--- | ||
+id: component-specs-zh-CN | ||
+title: 组件的规范和生命周期 | ||
+permalink: component-specs-zh-CN.html | ||
+prev: component-api-zh-CN.html | ||
+next: tags-and-attributes-zh-CN.html | ||
+--- | ||
+ | ||
+## 组件规范(Specifications) | ||
+ | ||
+当调用 `React.createClass()` 创建一个组件类时,你应该提供一个包含有 `render` 方法以及可选的其他生命周期方法的 规范(Specifications)对象. | ||
+ | ||
+> 注意: | ||
+> | ||
+> 同样可以使用单纯的 JavaScript 类作为组件类. 这些类可以实现大多数相同的方法,虽然有一些不同.更多关于不同的信息,请阅读我们关于[ES6 classes](/react/docs/reusable-components.html#es6-classes)的文档. | ||
+ | ||
+### render | ||
+ | ||
+```javascript | ||
+ReactElement render() | ||
+``` | ||
+ | ||
+ `render()` 是必须的 | ||
+ | ||
+ 当被调用时,它应该检查 `this.props` 和 `this.state` 并返回单个子元素.这个子元素即可以是一个 对原生DOM的虚拟表达(比如 `<div />` 或 `React.DOM.div()`)也可以是其他你自定义的复合组件. | ||
+ | ||
+ 你也可以返回 `null` 或 `false` 来指示你不想要任何东西被渲染.幕后,React 渲染一个 `<noscript>` tag 来与我们当前的diffing算法协同工作.当返回 `null` 或 `false` ,`ReactDOM.findDOMNode(this)` 会返回 `null`. | ||
+ | ||
+ `render()` 函数应该是纯净的,意味着它不改变组件的状态,它在每次调用时返回相同的结果,并且它不读和写 DOM 或者其他方式与浏览器互动(例如,使用 `setTimeout`).如果你需要与浏览器互动,在 `componentDidMount()` 里执行你的工作,或者其他生命周期方法里.保持 `render()` 纯净使服务器渲染更实用并且让组件更容易被思考. | ||
+ | ||
+ | ||
+ ### getInitialState | ||
+ | ||
+ ```javascript | ||
+object getInitialState() | ||
+``` | ||
+ | ||
+当组件被挂载时调用一次.返回值会被用作为 `this.state` 的初始值. | ||
+ | ||
+ | ||
+### getDefaultProps | ||
+ | ||
+```javascript | ||
+object getDefaultProps() | ||
+``` | ||
+ | ||
+在类被创建时调用一次并被缓存.在这个mapping里的值会被设置给 `this.props` 如果父组件没有指定对应的 prop (例如 使用一个 `in` 检查). | ||
+ | ||
+这个方法在任何实例被创建之前调用,因此不能依赖于 `this.props`.另外,小心,任何被 `getDefaultProps()`返回的复杂对象会被跨实例共享,而不是被拷贝. | ||
+ | ||
+ | ||
+### propTypes | ||
+ | ||
+```javascript | ||
+object propTypes | ||
+``` | ||
+ | ||
+ `propTypes` 对象允许你验证传递到你的组建的 props.更多关于 `propTypes` 的信息,见 [Reusable Components](/react/docs/reusable-components.html). | ||
+ | ||
+ | ||
+### mixins | ||
+ | ||
+```javascript | ||
+array mixins | ||
+``` | ||
+ | ||
+ `mixins` 数组允许你用 mixins 来在多个组件间共享行为.更多关于 mixins 的信息,见 [Reusable Components](/react/docs/reusable-components.html). | ||
+ | ||
+ | ||
+### statics | ||
+ | ||
+```javascript | ||
+object statics | ||
+``` | ||
+ | ||
+`statics` 对象允许你定义可以在组件类上调用的静态方法.例如: | ||
+ | ||
+```javascript | ||
+var MyComponent = React.createClass({ | ||
+ statics: { | ||
+ customMethod: function(foo) { | ||
+ return foo === 'bar'; | ||
+ } | ||
+ }, | ||
+ render: function() { | ||
+ } | ||
+}); | ||
+ | ||
+MyComponent.customMethod('bar'); // true | ||
+``` | ||
+ | ||
+在这个块里定义的方法是 _static_,意味着你可以在任何组件实例被创建前运行他们,并且这些方法没有对你组件的 props 或 state 的访问权.如果你在静态方法里检查props的值,把调用者作为参数传入props给静态函数. | ||
+ | ||
+ | ||
+### displayName | ||
+ | ||
+```javascript | ||
+string displayName | ||
+``` | ||
+ | ||
+`displayName` 字符串被用在调试信息.JSX 自动设置这个值;见 [JSX in Depth](/react/docs/jsx-in-depth.html#the-transform). | ||
+ | ||
+ | ||
+## Lifecycle Methods | ||
+ | ||
+多种方法在组件生命周期的特定点上被执行. | ||
+ | ||
+ | ||
+### Mounting: componentWillMount | ||
+ | ||
+```javascript | ||
+void componentWillMount() | ||
+``` | ||
+ | ||
+被调用一次,即在客户端也在服务端,在最初的渲染发生之前 立即被调用.如果你在这个方法里调用 `setState` , `render()` 将会看到更新的 state 并不论state的变化只执行一次. | ||
+ | ||
+ | ||
+### Mounting: componentDidMount | ||
+ | ||
+```javascript | ||
+void componentDidMount() | ||
+``` | ||
+ | ||
+被调用一次,只在客户端(不在服务端),在最初的渲染发生之后 立即被调用.在生命周期的这个点上,你可以访问任何对你的子级的refs (比如 访问底层的DOM表达).子组件的 `componentDidMount()` 方法在父组件之前被调用. | ||
+ | ||
+如果你想与其他 JavaScript 框架整合,用 `setTimeout` 或 `setInterval` 设置timers,或者发送 AJAX 请求,执行这些操作在此方法中. | ||
+ | ||
+ | ||
+### Updating: componentWillReceiveProps | ||
+ | ||
+```javascript | ||
+void componentWillReceiveProps( | ||
+ object nextProps | ||
+) | ||
+``` | ||
+ | ||
+当一个组件收到新的props时被调用.这个方法不会为最初的渲染调用. | ||
+ | ||
+使用它作为响应 prop 转换的时机(在`render()` 被用 `this.setState()` 更新state调用 之前) .旧的 props 可以通过 `this.props` 访问. 在这个函数里调用 `this.setState()` 不会触发任何额外的渲染. | ||
+ | ||
+```javascript | ||
+componentWillReceiveProps: function(nextProps) { | ||
+ this.setState({ | ||
+ likesIncreasing: nextProps.likeCount > this.props.likeCount | ||
+ }); | ||
+} | ||
+``` | ||
+ | ||
+> 注意: | ||
+> | ||
+> 并没有类似的 `componentWillReceiveState` 方法. 一个即将到来的 prop 转变可能会导致一个 state 变化,但是反之不是. 如果你需要实现一个对 state 变化相应的操作,使用 `componentWillUpdate`. | ||
+ | ||
+ | ||
+### Updating: shouldComponentUpdate | ||
+ | ||
+```javascript | ||
+boolean shouldComponentUpdate( | ||
+ object nextProps, object nextState | ||
+) | ||
+``` | ||
+ | ||
+当新的props或者state被收到,在渲染前被调用.这个方法不会在最初的渲染或者 `forceUpdate` 时被调用. | ||
+ | ||
+使用此方法作为一个 `return false` 的时机,当你确定新的 props 和 state 的转换不需要组件更新时. | ||
+ | ||
+```javascript | ||
+shouldComponentUpdate: function(nextProps, nextState) { | ||
+ return nextProps.id !== this.props.id; | ||
+} | ||
+``` | ||
+ | ||
+如果 `shouldComponentUpdate` 返回false, `render()` 会在下次state变化前被完全跳过. 另外,`componentWillUpdate` 和 `componentDidUpdate` 将不会被调用. | ||
+ | ||
+默认情况下, `shouldComponentUpdate` 总是返回 `true` 来阻止当 `state` 突变时的细微bug,但是如果你仔细的把 `state` 作为不变量对待并只从 `render()`里的 `props` 和 `state`读,你就可以用一个比较旧的props和state与他们的替换者的实现来重写 `shouldComponentUpdate`. | ||
+ | ||
+如果性能是瓶颈,尤其是随着成百上千的组件,使用 `shouldComponentUpdate` 来加速你的app. | ||
+ | ||
+ | ||
+### Updating: componentWillUpdate | ||
+ | ||
+```javascript | ||
+void componentWillUpdate( | ||
+ object nextProps, object nextState | ||
+) | ||
+``` | ||
+ | ||
+当新的props或者state被接受时,在渲染前被立即调用.这个方法不会被初始渲染调用. | ||
+ | ||
+使用这个方法作为 在更新发生前执行一些准备 的时机. | ||
+ | ||
+> Note: | ||
+> | ||
+> 你 *不能* 在这个方法里使用 `this.setState()` .如果你需要响应一个prop变化来更新state,使用 `componentWillReceiveProps` 来替代. | ||
+ | ||
+ | ||
+### Updating: componentDidUpdate | ||
+ | ||
+```javascript | ||
+void componentDidUpdate( | ||
+ object prevProps, object prevState | ||
+) | ||
+``` | ||
+ | ||
+在组件的更新被刷新到DOM后立即被调用.这个方法不会被初始渲染调用. | ||
+ | ||
+使用这个方法作为 当组件被更新后在DOM上操作 的时机. | ||
+ | ||
+ | ||
+### Unmounting: componentWillUnmount | ||
+ | ||
+```javascript | ||
+void componentWillUnmount() | ||
+``` | ||
+ | ||
+在组件被从DOM卸载 前 被立即调用. | ||
+ | ||
+在这个方法里执行一些必要的清理操作,比如无效化 timers 或者清理任何被 `componentDidMount` 创建的DOM元素. |
90
docs/docs/ref-04-tags-and-attributes.zh-CN.md
@@ -0,0 +1,90 @@ | ||
+--- | ||
+id: tags-and-attributes-zh-CN | ||
+title: Tags和属性 | ||
+permalink: tags-and-attributes-zh-CN.html | ||
+prev: component-specs-zh-CN.html | ||
+next: events-zh-CN.html | ||
+--- | ||
+ | ||
+## 支持的Tags | ||
+ | ||
+React试着支持所有常见的元素.如果你需要一个没有列在这里的元素,请 [file an issue](https://github.com/facebook/react/issues/new). | ||
+ | ||
+### HTML 元素 | ||
+ | ||
+下面的HTML是被支持的: | ||
+ | ||
+``` | ||
+a abbr address area article aside audio b base bdi bdo big blockquote body br | ||
+button canvas caption cite code col colgroup data datalist dd del details dfn | ||
+dialog div dl dt em embed fieldset figcaption figure footer form h1 h2 h3 h4 h5 | ||
+h6 head header hr html i iframe img input ins kbd keygen label legend li link | ||
+main map mark menu menuitem meta meter nav noscript object ol optgroup option | ||
+output p param picture pre progress q rp rt ruby s samp script section select | ||
+small source span strong style sub summary sup table tbody td textarea tfoot th | ||
+thead time title tr track u ul var video wbr | ||
+``` | ||
+ | ||
+### SVG 元素 | ||
+ | ||
+下面的 SVG 元素是被支持的: | ||
+ | ||
+``` | ||
+circle clipPath defs ellipse g line linearGradient mask path pattern polygon polyline | ||
+radialGradient rect stop svg text tspan | ||
+``` | ||
+ | ||
+你也许对 [react-art](https://github.com/facebook/react-art)有兴趣,一个让React绘制Canvas, SVG, 或者 VML (for IE8) 的绘制库. | ||
+ | ||
+ | ||
+## 支持的属性 | ||
+ | ||
+React支持所有的 `data-*` 和 `aria-*` 以及下列的属性. | ||
+ | ||
+> 注意: | ||
+> | ||
+> 所有的属性都是 camel-cased ,`class` 和 `for` 分别是 `className` 和 `htmlFor`,来符合DOM API 规范. | ||
+ | ||
+关于事件的列表,见 [Supported Events](/react/docs/events.html). | ||
+ | ||
+### HTML 属性 | ||
+ | ||
+下面的标准属性是被支持的: | ||
+ | ||
+``` | ||
+accept acceptCharset accessKey action allowFullScreen allowTransparency alt | ||
+async autoComplete autoFocus autoPlay capture cellPadding cellSpacing charSet | ||
+challenge checked classID className cols colSpan content contentEditable contextMenu | ||
+controls coords crossOrigin data dateTime defer dir disabled download draggable | ||
+encType form formAction formEncType formMethod formNoValidate formTarget frameBorder | ||
+headers height hidden high href hrefLang htmlFor httpEquiv icon id inputMode | ||
+keyParams keyType label lang list loop low manifest marginHeight marginWidth max | ||
+maxLength media mediaGroup method min minLength multiple muted name noValidate open | ||
+optimum pattern placeholder poster preload radioGroup readOnly rel required role | ||
+rows rowSpan sandbox scope scoped scrolling seamless selected shape size sizes | ||
+span spellCheck src srcDoc srcSet start step style summary tabIndex target title | ||
+type useMap value width wmode wrap | ||
+``` | ||
+ | ||
+另外,支持下面的非标准属性: | ||
+ | ||
+- `autoCapitalize autoCorrect` for Mobile Safari. | ||
+- `property` for [Open Graph](http://ogp.me/) meta tags. | ||
+- `itemProp itemScope itemType itemRef itemID` for [HTML5 microdata](http://schema.org/docs/gs.html). | ||
+- `unselectable` for Internet Explorer. | ||
+- `results autoSave` for WebKit/Blink input fields of type `search`. | ||
+ | ||
+同样有React规范的属性 `dangerouslySetInnerHTML` ([more here](/react/docs/special-non-dom-attributes.html)),用于直接插入HTML字符串到组件里. | ||
+ | ||
+### SVG 属性 | ||
+ | ||
+``` | ||
+clipPath cx cy d dx dy fill fillOpacity fontFamily | ||
+fontSize fx fy gradientTransform gradientUnits markerEnd | ||
+markerMid markerStart offset opacity patternContentUnits | ||
+patternUnits points preserveAspectRatio r rx ry spreadMethod | ||
+stopColor stopOpacity stroke strokeDasharray strokeLinecap | ||
+strokeOpacity strokeWidth textAnchor transform version | ||
+viewBox x1 x2 x xlinkActuate xlinkArcrole xlinkHref xlinkRole | ||
+xlinkShow xlinkTitle xlinkType xmlBase xmlLang xmlSpace y1 y2 y | ||
+``` |
50
docs/docs/ref-05-events.zh-CN.md
16
docs/docs/ref-06-dom-differences.zh-CN.md
@@ -0,0 +1,16 @@ | ||
+--- | ||
+id: dom-differences-zh-CN | ||
+title: DOM 的不同之处 | ||
+permalink: dom-differences-zh-CN.html | ||
+prev: events-zh-CN.html | ||
+next: special-non-dom-attributes-zh-CN.html | ||
+--- | ||
+ | ||
+React 实现了一个浏览器无关的 事件和DOM 系统,原因是为了性能和跨浏览器的兼容性.我们利用这个机会来清理了一些浏览器DOM实现的一些粗糙边缘. | ||
+ | ||
+* 所有的 DOM properties 和 attributes (包括事件处理器) 都应该 camelCased 来保持和标准的 JavaScript 风格一致.我们在这里故意打破了这个规范是因为规范是不一致的. **然而**,`data-*` 和 `aria-*` attributes [conform to the specs](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes#data-*) 应该只 lower-cased. | ||
+* `style` attribute 接受 camelCased properties 的JavaScript对象 而不是一个CSS字符串. 这保持了和 DOM `style` JavaScript property 的一致,更有效率,而且阻止了XSS安全漏洞. | ||
+* 因为 `class` 和 `for` 是 JavaScript的保留字,内建[DOM nodes](http://javascript.info/tutorial/dom-nodes)的JSX元素 应该分别使用 attribute名`className` 和 `htmlFor` ,(比如 `<div className="foo" />`).自定义元素应该直接使用 `class` 和 `for` (例如. `<my-tag class="foo" />` ). | ||
+* 所有事件对象遵循 W3C 规范,所有事件(包括提交)正确按W3C规范冒泡.详见[Event System](/react/docs/events.html). | ||
+* `onChange` 事件表现的像你期望的那样:不论何时一个表单域改变了这个事件就会激发,而不是模糊的不一致.我们故意打破了已有浏览器的行为,因为 `onChange` 对于他的行为来说用词不当,并且React依赖于这个事件来实时响应用户的输入.详见[Forms](/react/docs/forms.html). | ||
+* 表单 输入attributes 类似 `value` 和 `checked`,就像`textarea`一样[More here](/react/docs/forms.html). |
13
docs/docs/ref-07-special-non-dom-attributes.zh-CN.md
@@ -0,0 +1,13 @@ | ||
+--- | ||
+id: special-non-dom-attributes-zh-CN | ||
+title: 特殊的 Non-DOM Attributes | ||
+permalink: special-non-dom-attributes-zh-CN.html | ||
+prev: dom-differences-zh-CN.html | ||
+next: reconciliation-zh-CN.html | ||
+--- | ||
+ | ||
+和 [DOM 的不同之处](/react/docs/dom-differences-zh-CN.html)相比, React 提供了一些不存在于DOM的 attributes . | ||
+ | ||
+- `key`: 一个可选的.独特的标识.当你的组件穿梭于 `render` 的pass,它也许会因为diff算法被摧毁和重建.赋予它一个持久的key保证这个component可达.详见 [这里](/react/docs/multiple-components.html#dynamic-children). | ||
+- `ref`: 见 [这里](/react/docs/more-about-refs.html). | ||
+- `dangerouslySetInnerHTML`: 提供了直接插入raw HTML的能力,主要是为了与操纵DOM字符串的库协作.详见 [这里](/react/tips/dangerously-set-inner-html.html). |
193
docs/docs/ref-10-glossary.zh-CN.md
@@ -0,0 +1,193 @@ | ||
+--- | ||
+id: glossary-zh-CN | ||
+title: React (虚拟) DOM 术语 | ||
+permalink: glossary-zh-CN.html | ||
+prev: webcomponents-zh-CN.html | ||
+--- | ||
+ | ||
+在 React 的术语中,有五个要重点区分的核心类型: | ||
+ | ||
+- [ReactElement / ReactElement Factory](#react-elements) | ||
+- [ReactNode](#react-nodes) | ||
+- [ReactComponent / ReactComponent Class](#react-components) | ||
+ | ||
+## React Elements(React 元素) | ||
+ | ||
+React里的首要类型是 `ReactElement`.它有四个 properties:`type`, `props`, `key` 和 `ref`.它没有方法,在 prototype 上什么也没有. | ||
+ | ||
+你可以通过 `React.createElement` 来创建这些对象. | ||
+ | ||
+```javascript | ||
+var root = React.createElement('div'); | ||
+``` | ||
+ | ||
+要渲染一个新的树到DOM上,你创建 `ReactElement`s 并传递他们到 `ReactDOM.render` 伴随着一个标准的 DOM `Element` (`HTMLElement` 或 `SVGElement`).`ReactElement`s 不要与 DOM `Element`s 混淆.`ReactElement` 是一个轻的,有状态的,不可变的,虚拟的DOM `Element` 的表达.它是一个虚拟 DOM. | ||
+ | ||
+```javascript | ||
+ReactDOM.render(root, document.getElementById('example')); | ||
+``` | ||
+ | ||
+要给一个DOM元素添加 properties,传递一个properties 对象作为第二个参数,第三个参数传递子级. | ||
+ | ||
+```javascript | ||
+var child = React.createElement('li', null, 'Text Content'); | ||
+var root = React.createElement('ul', { className: 'my-list' }, child); | ||
+ReactDOM.render(root, document.getElementById('example')); | ||
+``` | ||
+ | ||
+如果你使用 React JSX,这些`ReactElement`s 已经为你创建了.所以 这是等价的: | ||
+ | ||
+```javascript | ||
+var root = <ul className="my-list"> | ||
+ <li>Text Content</li> | ||
+ </ul>; | ||
+ReactDOM.render(root, document.getElementById('example')); | ||
+``` | ||
+ | ||
+### Factories(工厂) | ||
+ | ||
+`ReactElement`-工厂 是一个产生特定 `type` property的 `ReactElement` 的函数.React有一个为你内建的辅助工具来创建工厂.它想这样起作用: | ||
+ | ||
+```javascript | ||
+function createFactory(type) { | ||
+ return React.createElement.bind(null, type); | ||
+} | ||
+``` | ||
+ | ||
+它允许你创建一个方便的速记 来代替每次输入 `React.createElement('div')` . | ||
+ | ||
+```javascript | ||
+var div = React.createFactory('div'); | ||
+var root = div({ className: 'my-div' }); | ||
+ReactDOM.render(root, document.getElementById('example')); | ||
+``` | ||
+ | ||
+React 已经具备用于常用 HTML tags的内建工厂 | ||
+ | ||
+```javascript | ||
+var root = React.DOM.ul({ className: 'my-list' }, | ||
+ React.DOM.li(null, 'Text Content') | ||
+ ); | ||
+``` | ||
+ | ||
+如果你使用JSX 你没有必要使用工厂.JSX已经为创建 `ReactElement`s 提供了一个 方便的速记. | ||
+ | ||
+ | ||
+## React Nodes | ||
+ | ||
+一个 `ReactNode` 可以是: | ||
+ | ||
+- `ReactElement` | ||
+- `string` (aka `ReactText`) | ||
+- `number` (aka `ReactText`) | ||
+- Array of `ReactNode`s (aka `ReactFragment`) | ||
+ | ||
+他们被用作其他`ReactElement`s的properties来表示子级.事实上他们创建了一个 `ReactElement`s 的树. | ||
+ | ||
+ | ||
+## React Components | ||
+ | ||
+你可以使用 React只使用`ReactElement`s 但是要真正利用React,你将要使用 `ReactComponent`s 来创建内嵌 state 的封装. | ||
+ | ||
+一个 `ReactComponent` 类就是一个 JavaScript 类 (或者 "constructor function"). | ||
+ | ||
+```javascript | ||
+var MyComponent = React.createClass({ | ||
+ render: function() { | ||
+ ... | ||
+ } | ||
+}); | ||
+``` | ||
+ | ||
+当这个构造函数被调用,期望返回一个至少有一个 `render` 方法的对象.这个对象被称为一个 `ReactComponent`. | ||
+ | ||
+```javascript | ||
+var component = new MyComponent(props); // never do this | ||
+``` | ||
+ | ||
+与测试不同,你可能通常 *绝不会* 亲自调用这个构造函数.React 为你调用它. | ||
+ | ||
+作为替代,你传递 `ReactComponent` 类到 `createElement`,你得到一个 `ReactElement`. | ||
+ | ||
+```javascript | ||
+var element = React.createElement(MyComponent); | ||
+``` | ||
+ | ||
+或者用 JSX: | ||
+ | ||
+```javascript | ||
+var element = <MyComponent />; | ||
+``` | ||
+ | ||
+当这个被传给 `ReactDOM.render`,React 会为你调用构造函数并创建一个 `ReactComponent`,返回给你. | ||
+ | ||
+```javascript | ||
+var component = ReactDOM.render(element, document.getElementById('example')); | ||
+``` | ||
+ | ||
+如果你保持用相同类型的 `ReactElement` 和相同的DOM `Element`容器调用 `ReactDOM.render` ,它总是会返回相同的实例.这个实例是状态化的. | ||
+ | ||
+```javascript | ||
+var componentA = ReactDOM.render(<MyComponent />, document.getElementById('example')); | ||
+var componentB = ReactDOM.render(<MyComponent />, document.getElementById('example')); | ||
+componentA === componentB; // true | ||
+``` | ||
+ | ||
+这就是为什么你不应该构造你自己的实例.作为替代,`ReactElement` 在它被构造以前 是一个虚拟的 `ReactComponent`.一个老的和新的`ReactElement` 可以被比较来判断 一个新的 `ReactComponent` 实例是否需要被创建或者已经存在的是否应该被重用. | ||
+ | ||
+ `ReactComponent` 的 `render` 方法被期望返回另一个 `ReactElement`.这允许这些组件被结构化.最后,渲染分解为 带着一个 `string` tag的`ReactElement`,它实例化一个 DOM `Element` 实例并把它插入document里. | ||
+ | ||
+ | ||
+## Formal Type Definitions | ||
+ | ||
+### Entry Point | ||
+ | ||
+``` | ||
+ReactDOM.render = (ReactElement, HTMLElement | SVGElement) => ReactComponent; | ||
+``` | ||
+ | ||
+### Nodes and Elements | ||
+ | ||
+``` | ||
+type ReactNode = ReactElement | ReactFragment | ReactText; | ||
+ | ||
+type ReactElement = ReactComponentElement | ReactDOMElement; | ||
+ | ||
+type ReactDOMElement = { | ||
+ type : string, | ||
+ props : { | ||
+ children : ReactNodeList, | ||
+ className : string, | ||
+ etc. | ||
+ }, | ||
+ key : string | boolean | number | null, | ||
+ ref : string | null | ||
+}; | ||
+ | ||
+type ReactComponentElement<TProps> = { | ||
+ type : ReactClass<TProps>, | ||
+ props : TProps, | ||
+ key : string | boolean | number | null, | ||
+ ref : string | null | ||
+}; | ||
+ | ||
+type ReactFragment = Array<ReactNode | ReactEmpty>; | ||
+ | ||
+type ReactNodeList = ReactNode | ReactEmpty; | ||
+ | ||
+type ReactText = string | number; | ||
+ | ||
+type ReactEmpty = null | undefined | boolean; | ||
+``` | ||
+ | ||
+### Classes and Components | ||
+ | ||
+``` | ||
+type ReactClass<TProps> = (TProps) => ReactComponent<TProps>; | ||
+ | ||
+type ReactComponent<TProps> = { | ||
+ props : TProps, | ||
+ render : () => ReactElement | ||
+}; | ||
+``` | ||
+ |
2
docs/docs/thinking-in-react.zh-CN.md
173
docs/docs/videos.zh-CN.md
@@ -0,0 +1,173 @@ | ||
+--- | ||
+id: videos-zh-CN | ||
+title: 视频 | ||
+permalink: videos-zh-CN.html | ||
+prev: conferences-zh-CN.html | ||
+next: complementary-tools-zh-CN.html | ||
+--- | ||
+ | ||
+### Rethinking best practices - JSConf.eu | ||
+ | ||
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/embed/x7cQ3mrcKaY" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+"在 Facebook 和 Instagram, 我们正在努力挑战React在web上能达到的极限。我的讲话会从对框架的简单介绍开始,然后深入三个有争议的话题:扔掉模板的概念并用JavaScript构建views, 当数据改变 “re-rendering” 你的整个应用,以及一个DOM和events的轻量级实现。" -- [Pete Hunt](http://www.petehunt.net/) | ||
+ | ||
+* * * | ||
+ | ||
+### Thinking in react - tagtree.tv | ||
+ | ||
+一个 [tagtree.tv](http://tagtree.tv/) 传达 [Thinking in React](/react/docs/thinking-in-react.html) 原则的视频 在构建一个简单app时。 | ||
+<figure>[](http://tagtree.tv/thinking-in-react)</figure> | ||
+ | ||
+* * * | ||
+ | ||
+### Secrets of the Virtual DOM - MtnWest JS | ||
+ | ||
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/embed/h3KksH8gfcQ" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+"在这次讲座里,我会讨论为什么我们构建了一个虚拟 DOM,它比起其他系统如何,以及它与未来浏览器技术的关系。" -- [Pete Hunt](http://www.petehunt.net/) | ||
+ | ||
+* * * | ||
+ | ||
+### Going big with React ### | ||
+ | ||
+"理论上,所有的JS框架都大有可为:干净的实现,快速的代码设计,完美的执行。但是当你压力测试时Javascript会怎样?当你丢进6MB的代码时会怎样?在这次演讲中,我们会探究React在高压环境下如何表现,以及它如何帮助我们的团队在大规模时构建安全代码。 " | ||
+<figure>[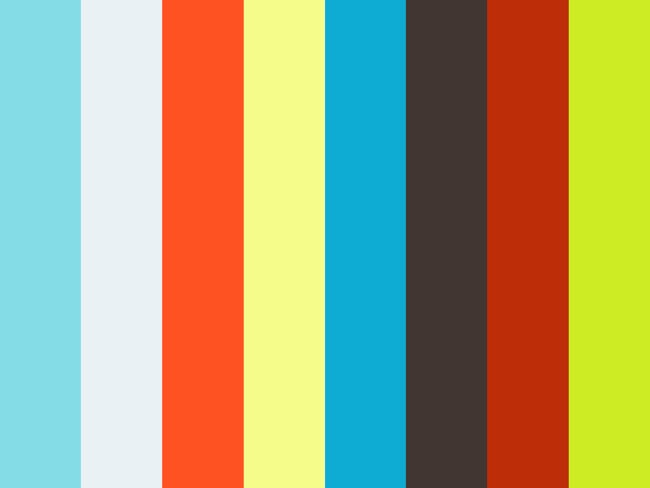](https://skillsmatter.com/skillscasts/5429-going-big-with-react#video)</figure> | ||
+ | ||
+* * * | ||
+ | ||
+### CodeWinds | ||
+ | ||
+[Pete Hunt](http://www.petehunt.net/) 与 [Jeff Barczewski](http://jeff.barczewski.com/) 在 CodeWinds Episode 4 上关于 React 的谈话. | ||
+<figure>[](http://codewinds.com/4)</figure> | ||
+ | ||
+<table width="100%"><tr><td> | ||
+02:08 - 什么是React,为什么我们用它?<br /> | ||
+03:08 - ClojureScript 和 React 的共生关系<br /> | ||
+04:54 - React 的历史以及为什么它被创造<br /> | ||
+09:43 - 用React更新Web页面,而不绑定数据<br /> | ||
+13:11 - 用虚拟DOM来改变浏览器DOM<br /> | ||
+13:57 - 用React编程,绘制目标HTML,canvas和其他<br /> | ||
+16:45 - 和设计师一起工作,对比于Ember 和 AngularJS<br /> | ||
+21:45 - JSX编译器桥接HTML和 React javascript<br /> | ||
+23:50 - React的自动绑定JSX以及浏览器内工具<br /> | ||
+24:50 - 用React工作的提示和技巧,入门<br /> | ||
+</td><td> | ||
+27:17 - 在服务器端用Node.js渲染HTML。后端渲染<br /> | ||
+29:20 - React在Facebook通过优胜劣汰进化<br /> | ||
+30:15 - 用web sockets,在服务器端和客户端持有状态的想法持有<br /> | ||
+32:05 - 多用户React - 用 Firebase 分布式共享可变状态<br /> | ||
+33:03 - 用状态转换,事件重放来更好的调式React<br /> | ||
+34:08 - 来自Web组件的不同之处<br /> | ||
+34:25 - 使用React的著名公司<br /> | ||
+35:16 - 一个React的后端插件可以用来创建PDF吗?<br /> | ||
+36:30 - React的未来,下一步是什么?<br /> | ||
+39:38 - 贡献和获得帮助<br /> | ||
+</td></tr></table> | ||
+ | ||
+[Read the episode notes](http://codewinds.com/4) | ||
+ | ||
+* * * | ||
+ | ||
+### JavaScript Jabber | ||
+ | ||
+[Pete Hunt](http://www.petehunt.net/) 和 [Jordan Walke](https://github.com/jordwalke) 在 JavaScript Jabber 73 上关于React的谈话. | ||
+<figure>[](http://javascriptjabber.com/073-jsj-react-with-pete-hunt-and-jordan-walke/#content)</figure> | ||
+ | ||
+<table width="100%"><tr><td> | ||
+01:34 – Pete Hunt 介绍<br /> | ||
+02:45 – Jordan Walke 介绍<br /> | ||
+04:15 – React<br /> | ||
+06:38 – 60 帧每秒<br /> | ||
+09:34 – 数据绑定<br /> | ||
+12:31 – 性能<br /> | ||
+17:39 – Diffing 算法<br /> | ||
+19:36 – DOM 操纵 | ||
+</td><td> | ||
+23:06 – 支持 node.js<br /> | ||
+24:03 – rendr<br /> | ||
+26:02 – JSX<br /> | ||
+30:31 – requestAnimationFrame<br /> | ||
+34:15 – React 和应用<br /> | ||
+38:12 – React 用户 Khan Academy<br /> | ||
+39:53 – 使其工作 | ||
+</td></tr></table> | ||
+ | ||
+[Read the full transcript](http://javascriptjabber.com/073-jsj-react-with-pete-hunt-and-jordan-walke/) | ||
+ | ||
+* * * | ||
+ | ||
+### Introduction to React.js - Facebook Seattle | ||
+ | ||
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/embed/XxVg_s8xAms" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+由 [Tom Occhino](http://tomocchino.com/) 和 [Jordan Walke](https://github.com/jordwalke) | ||
+ | ||
+* * * | ||
+ | ||
+### Backbone + React + Middleman Screencast | ||
+<iframe width="650" height="488" src="https://www.youtube-nocookie.com/embed/iul1fWHVU6A" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+Backbone 是一个在用React实现 REST API 接口的极好方法。这个屏博展示了用 [Backbone-React-Component](https://github.com/magalhas/backbone-react-component)如何整合两者. Middleman 是在本例中使用的框架但很容易被替换成其他框架。对此可支持的template可以在[这里](https://github.com/jbhatab/middleman-backbone-react-template) 找到. -- [Open Minded Innovations](http://www.openmindedinnovations.com/) | ||
+ | ||
+* * * | ||
+ | ||
+### Developing User Interfaces With React - Super VanJS | ||
+ | ||
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/embed/1OeXsL5mr4g" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+来自 [Steven Luscher](https://github.com/steveluscher) | ||
+ | ||
+* * * | ||
+ | ||
+### Introduction to React - LAWebSpeed meetup | ||
+ | ||
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/embed/SMMRJif5QW0" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+来自 [Stoyan Stefanov](http://www.phpied.com/) | ||
+ | ||
+* * * | ||
+ | ||
+### React, or how to make life simpler - FrontEnd Dev Conf '14 | ||
+ | ||
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/embed/YJNUK0EA_Jo" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+**俄语** by [Alexander Solovyov](http://solovyov.net/) | ||
+ | ||
+* * * | ||
+ | ||
+### "Functional DOM programming" - Meteor DevShop 11 | ||
+ | ||
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/embed/qqVbr_LaCIo" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+* * * | ||
+ | ||
+### "Rethinking Web App Development at Facebook" - Facebook F8 Conference 2014 | ||
+ | ||
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/embed/nYkdrAPrdcw" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+* * * | ||
+ | ||
+### React and Flux: Building Applications with a Unidirectional Data Flow - Forward JS 2014 | ||
+ | ||
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/embed/i__969noyAM" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+Facebook 工程师 [Bill Fisher](https://twitter.com/fisherwebdev) 和 [Jing Chen](https://twitter.com/jingc) 谈论 Flux 和 React, 以及如何使用单向数据流的程序架构清理他们的代码 . | ||
+ | ||
+* * * | ||
+ | ||
+### Server-Side Rendering of Isomorphic Apps at SoundCloud | ||
+ | ||
+<iframe src="https://player.vimeo.com/video/108488724" width="650" height="365" frameborder="0" webkitallowfullscreen mozallowfullscreen allowfullscreen></iframe> | ||
+ | ||
+来自 [Andres Suarez](https://github.com/zertosh) 的演练,关于 [SoundCloud](https://developers.soundcloud.com/blog/) 如何使用 React 和 Flux 在服务器端渲染. | ||
+ | ||
+[幻灯片和示例代码](https://github.com/zertosh/ssr-demo-kit) | ||
+ | ||
+* * * | ||
+ | ||
+### Introducing React Native (+Playlist) - React.js Conf 2015 | ||
+ | ||
+<iframe width="650" height="366" src="https://www.youtube-nocookie.com/embed/KVZ-P-ZI6W4?list=PLb0IAmt7-GS1cbw4qonlQztYV1TAW0sCr" frameborder="0" allowfullscreen></iframe> | ||
+ | ||
+[Tom Occhino](https://twitter.com/tomocchino) 回顾了React的过去和现在,在2015年。梳理了下一步要做什么。 |
0 comments on commit
badc15e