Permalink
Please sign in to comment.
Showing
with
867 additions
and 1,025 deletions.
- +2 −0 .gitignore
- +100 −116 10-Steps-To-Plan-Better-So-You-Can-Write-Less-Code.md
- +154 −151 Algorithm-Missing-letters.md
- +40 −40 Algorithm-Style-Guide.md
- +41 −36 Back-End-Project-Resources.md
- +5 −5 Checkpoint-Counting-Cards.md
- +3 −3 Checkpoint-Golf-Code.md
- +3 −3 Checkpoint-Profile-Lookup.md
- +4 −4 Checkpoint-Record-Collection.md
- +1 −1 Checkpoint-Shopping-List.md
- +6 −6 Checkpoint-Stand-In-Line.md
- +3 −3 Checkpoint-Word-Blanks.md
- +7 −7 Contributions-Guide---with-Typo-Demo.md
- +9 −11 Frontend-file-structure.md
- +33 −31 Guide-to-Back-End-Projects-Table-of-Contents.md
- +120 −97 How-to-get-the-MEAN-stack-running-locally-on-OSX.md
- +9 −9 Intro-to-Yeoman-Angular-Fullstack-Back-End-Projects.md
- +241 −241 Map.md
- +2 −2 Start-Here.md
- +81 −94 Using-Github-Pages-for-your-front-end-development-projects.md
- +0 −77 bf-bouncer.md
- +3 −3 html-elements.md
- +0 −85 npm-debug.log
2
.gitignore
216
10-Steps-To-Plan-Better-So-You-Can-Write-Less-Code.md
@@ -1,116 +1,100 @@ | ||
-##Learn how to plan your future project! | ||
- | ||
-*Blog post written by BiancaMihai - [GitHub](https://github.com/biancamihai) / [Twitter](https://twitter.com/intent/user?screen_name=bubuslubu)* | ||
- | ||
-An ounce of preparation is worth a pound of cure. That's true in medicine, and that's definitely true in software development. | ||
- | ||
-Here's a structured 10-step workflow that will guide you through the app planning process, with the goal of saving you from writing a lot of unnecessary code. | ||
- | ||
-Together, we'll plan out a simple "To-do" single-page web app. We'll also plan for an API backend for a future mobile app. | ||
- | ||
-###1) Create our Trello board | ||
- | ||
-[Trello](https://trello.com/) is a fun, free way to break your planning and development process into small tasks that can be tracked. | ||
- | ||
-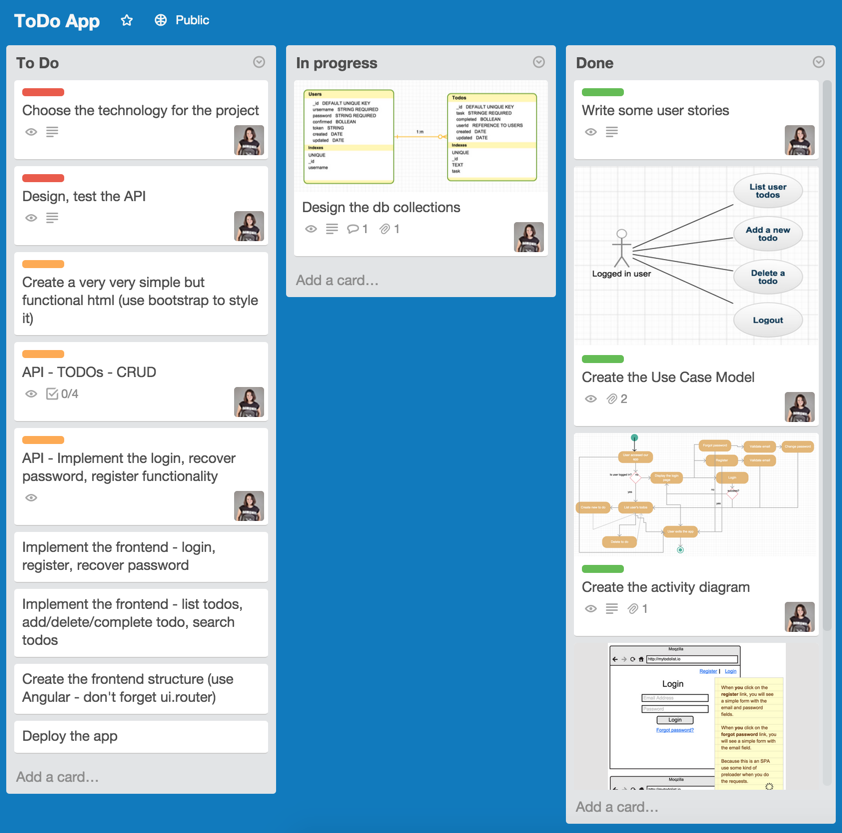 | ||
- | ||
- | ||
-[Here's what our Trello board will eventually look like](https://trello.com/b/O9MZcYyY/todo-app). I prefer to split my tasks into 3 columns (depending on the complexity of the project): | ||
- | ||
-* To Do - what is left to do | ||
-* In progress - tasks that people are currently working on | ||
-* Done - tasks that are done and ready for testing | ||
- | ||
-###2) Write user stories | ||
- | ||
-Here are some example user stories. These will guide how we think about our app's features and functionality. Note that they all follow a similar structure: as a [person] I can [do something]. | ||
- | ||
-* as a logged-in user I can see the list of my to-do's. | ||
-* as a logged-in user I can add a new to-do. | ||
-* as a logged-in user I can delete a to-do (only my to-do's - not other users'). | ||
-* as a logged-in user I can complete a to-do (only my to-do's - not other users'). | ||
-* as an anonymous user, I can register for a new account, recover my password, or log in to the app with an existing account. | ||
- | ||
-###3) Create our use case model | ||
- | ||
-Our use case model will help us visualize our user stories so we can better understand how to implement them. | ||
- | ||
-![Anonymous user case diagram] (https://lh5.googleusercontent.com/2v6iIMbCrLSKVfqttEToum7OA3YGQCBKWUHcSCB1KEbEcijXxQtKJKY6fhLXeecJiO27P4icOuPlkVc9_uNXolzlzNXOo_TPh09GZsAqRH-JISqPrpx0PZdtbHOr0RIuQUbTbaw) | ||
-![Authenticated user case diagram] (https://lh6.googleusercontent.com/3V6dVvAcyjqFkaOukimucYOX0CfwBBYNN9SvjmnVy40Pdhs4Wtrr34i3E-9pbV7tFsp4jHm77IFQvFupjq6OWyxqEgCzcQ995Ayh52Msczu6TfwKeNhL9PYHyxSgmPYA1TR6l6Q) | ||
- | ||
-###4) Create our activity diagram | ||
- | ||
-Our activity diagram will show the different paths our users can take through our app. | ||
- | ||
-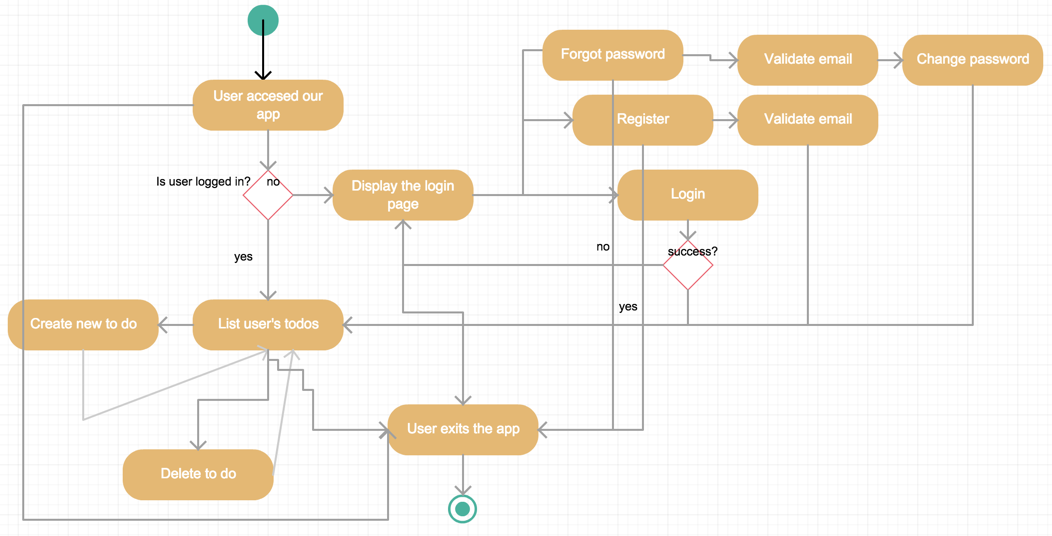 | ||
- | ||
-A user accesses our to-do application. | ||
-* If the user is not logged in she will see our login page. | ||
-* If she already has an account, she can log in. | ||
-* If she has an account, but she forgot her password, she can recover her password. | ||
-* If she doesn't have an account, she can create one. | ||
-* Both "create an account" and "recover my password" will require email validation. A user can log in to our application only after she has confirmed her email address. | ||
-* If she is logged in, she will see her to-do list (this can be empty if she hasn't added any to-dos yet). | ||
-* A logged in user: | ||
- * is able to see her tasks list | ||
- * is able to mark a task from her list as completed | ||
- * is able to search within her task list | ||
- * is able to delete a task from her list | ||
- * can logout. | ||
-* The user can exit the application at any time. | ||
- | ||
-###5) Create our mockups | ||
- | ||
-Our mockups show what our app should look like. It's much faster to iterate on a mockup than it is to do so on working code. | ||
- | ||
-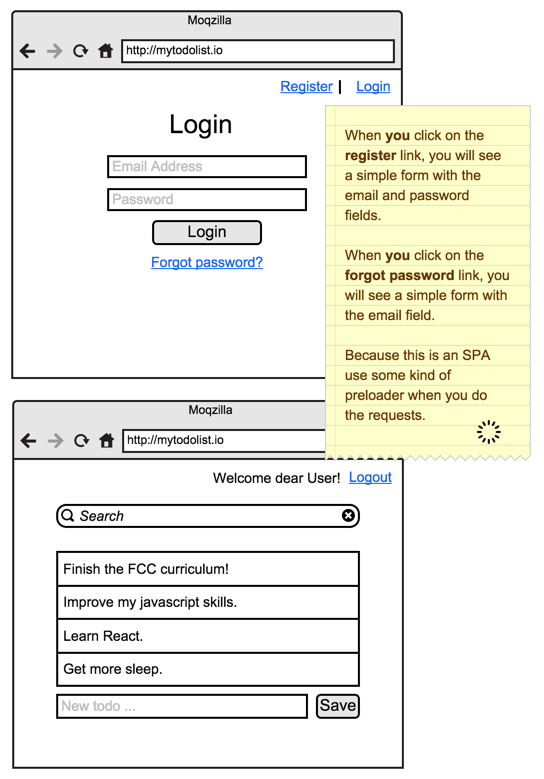 | ||
- | ||
-###6) Choose the right technologies for our project | ||
- | ||
-Because this is a single page app, we'll rely heavily - or in this case exclusively - on JavaScript. Let's use one of the most popular JavaScript stacks: the MEAN stack. One big benefit of the MEAN stack is that all of its components are free and open-source. There are also tons of resources available for learning the MEAN stack, and for debugging it when you inevitably encounter errors. | ||
- | ||
-You can have a [MEAN stack development environment](http://www.freecodecamp.com/challenges/Challenge-get-set-for-Back End Projects) up and running in the cloud in less than an hour, for free. | ||
- | ||
-Here are the components we'll use: | ||
-1. [MongoDB](http://mongodb.org/) for our database | ||
-2. [Node.js](http://nodejs.org/) and [Express.js](http://expressjs.com/) for implementing our API | ||
-3. [AngularJS](http://angularjs.org/), along with HTML and CSS (and Bootstrap) for our client-side application | ||
-4. [Mongoose](http://mongoosejs.com/) to connect our application to MongoDB | ||
- | ||
-###7) Design our database schema | ||
- | ||
-It's worth the effort to design a database schema, even for our simple application. | ||
- | ||
-We'll have two collections: our "Users" collection will house our user data, and our "ToDo" collection will house our tasks that need to be done. One user can have zero, one, or many tasks in her to-do list, so we will have a one-to-many (1:m) relationship between our two collections. | ||
- | ||
-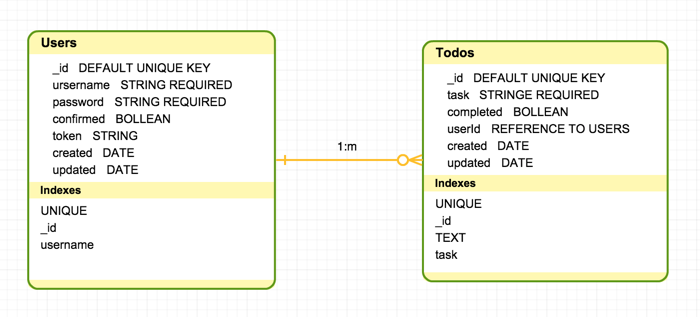 | ||
- | ||
-###8) Define our use cases | ||
- | ||
-1. What happens to the to-dos related to a user that deletes her account? When the user deletes her account the to-dos related to that user should also be deleted. | ||
-2. No to-do can be added without being attached to a confirmed user. | ||
-3. A to-do can only be deleted by its owner. | ||
-4. No user can be added with an empty username or password. | ||
-5. No to-do can be added with an empty task. | ||
- | ||
-Things to keep in mind: | ||
- | ||
-1. Use the Mongoose middleware to remove dependent documents like to-dos when a user deletes her account. | ||
-2. Use Mongoose validation rules on models to prevent empty fields from being added to our database. | ||
- | ||
-###9) Design and test our API | ||
- | ||
-I used a free product called Apiary [to document our API](http://docs.fcctodoapp.apiary.io/). | ||
- | ||
-Here's the syntax I used to [create this simple BluePrint](https://jsapi.apiary.io/apis/fcctodoapp.apib). | ||
- | ||
-I recommend you create an account and start playing with it. If you link your [GitHub](http://github.com/) account with Apiary, you can ensure your documentation always stays up to date. You'll also be able to test your data visually without the need for actually hitting your API endpoints. If you prefer to test your API from the command line, [here's an example of how to do this](http://docs.agendor.apiary.io/). | ||
- | ||
- | ||
-Later, once you've implemented your API with Node.js and Express.js, you'll just need to set your URL in Apiary. Then you can start testing your calls. Our current host url (http://fcctodoapp.apiblueprint.org/) will be replaced by your API's URL. | ||
- | ||
-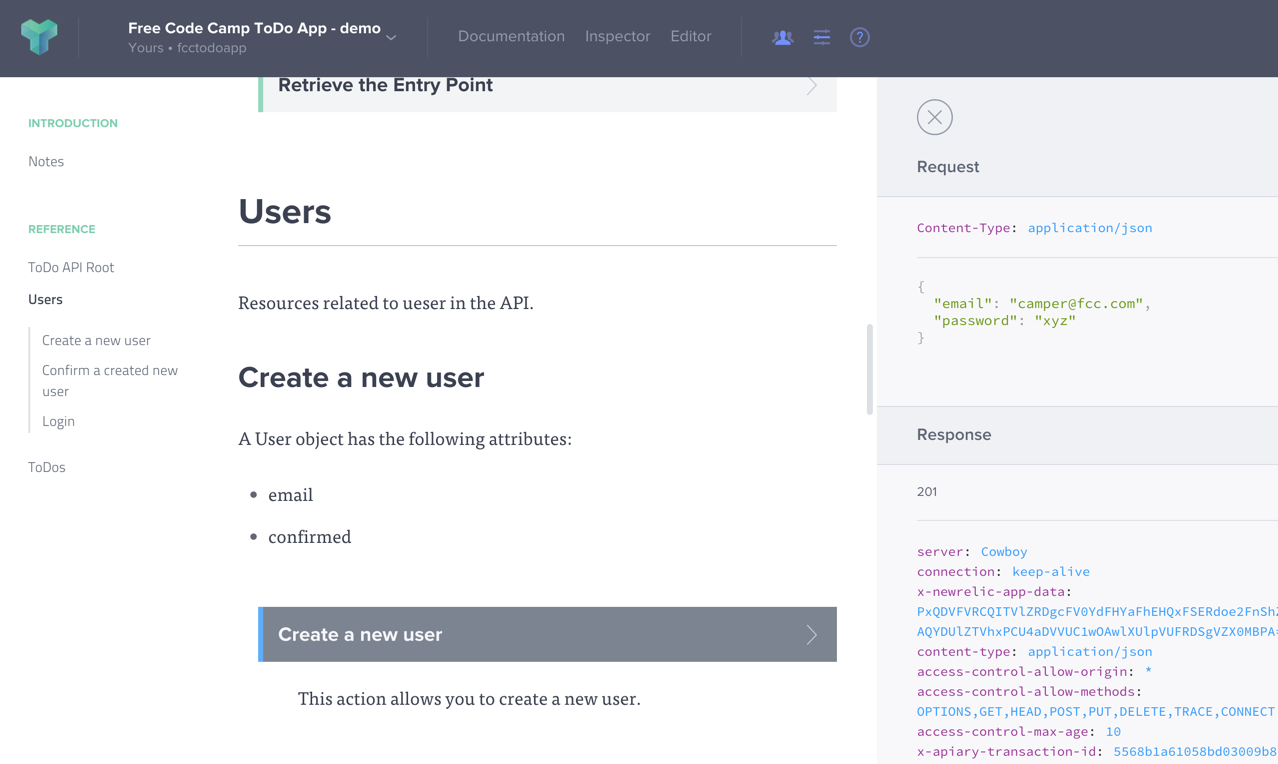 | ||
- | ||
-###10) Start writing code! | ||
- | ||
-This is the fun part, and it will take up most of your project's time. If you need help with this, join us and learn to code. | ||
+# Learn how to plan your future project! | ||
+_Blog post written by BiancaMihai - [GitHub](https://github.com/biancamihai) / [Twitter](https://twitter.com/intent/user?screen_name=bubuslubu)_ | ||
+ | ||
+An ounce of preparation is worth a pound of cure. That's true in medicine, and that's definitely true in software development. | ||
+ | ||
+Here's a structured 10-step workflow that will guide you through the app planning process, with the goal of saving you from writing a lot of unnecessary code. | ||
+ | ||
+Together, we'll plan out a simple "To-do" single-page web app. We'll also plan for an API backend for a future mobile app. | ||
+ | ||
+## 1) Create our Trello board | ||
+[Trello](https://trello.com/) is a fun, free way to break your planning and development process into small tasks that can be tracked. | ||
+ | ||
+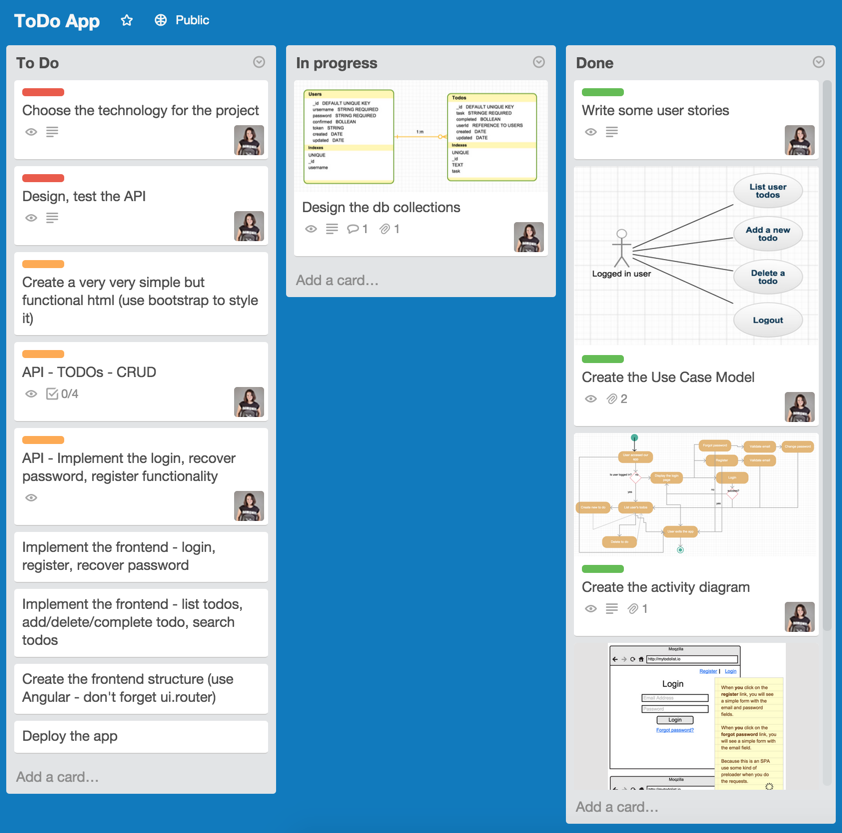 | ||
+ | ||
+[Here's what our Trello board will eventually look like](https://trello.com/b/O9MZcYyY/todo-app). I prefer to split my tasks into 3 columns (depending on the complexity of the project): | ||
+- To Do - what is left to do | ||
+- In progress - tasks that people are currently working on | ||
+- Done - tasks that are done and ready for testing | ||
+ | ||
+## 2) Write user stories | ||
+Here are some example user stories. These will guide how we think about our app's features and functionality. Note that they all follow a similar structure: as a [person] I can [do something]. | ||
+- as a logged-in user I can see the list of my to-do's. | ||
+- as a logged-in user I can add a new to-do. | ||
+- as a logged-in user I can delete a to-do (only my to-do's - not other users'). | ||
+- as a logged-in user I can complete a to-do (only my to-do's - not other users'). | ||
+- as an anonymous user, I can register for a new account, recover my password, or log in to the app with an existing account. | ||
+ | ||
+## 3) Create our use case model | ||
+Our use case model will help us visualize our user stories so we can better understand how to implement them. | ||
+ | ||
+![Anonymous user case diagram] ([https://lh5.googleusercontent.com/2v6iIMbCrLSKVfqttEToum7OA3YGQCBKWUHcSCB1KEbEcijXxQtKJKY6fhLXeecJiO27P4icOuPlkVc9_uNXolzlzNXOo_TPh09GZsAqRH-JISqPrpx0PZdtbHOr0RIuQUbTbaw](https://lh5.googleusercontent.com/2v6iIMbCrLSKVfqttEToum7OA3YGQCBKWUHcSCB1KEbEcijXxQtKJKY6fhLXeecJiO27P4icOuPlkVc9_uNXolzlzNXOo_TPh09GZsAqRH-JISqPrpx0PZdtbHOr0RIuQUbTbaw)) ![Authenticated user case diagram] ([https://lh6.googleusercontent.com/3V6dVvAcyjqFkaOukimucYOX0CfwBBYNN9SvjmnVy40Pdhs4Wtrr34i3E-9pbV7tFsp4jHm77IFQvFupjq6OWyxqEgCzcQ995Ayh52Msczu6TfwKeNhL9PYHyxSgmPYA1TR6l6Q](https://lh6.googleusercontent.com/3V6dVvAcyjqFkaOukimucYOX0CfwBBYNN9SvjmnVy40Pdhs4Wtrr34i3E-9pbV7tFsp4jHm77IFQvFupjq6OWyxqEgCzcQ995Ayh52Msczu6TfwKeNhL9PYHyxSgmPYA1TR6l6Q)) | ||
+ | ||
+## 4) Create our activity diagram | ||
+Our activity diagram will show the different paths our users can take through our app. | ||
+ | ||
+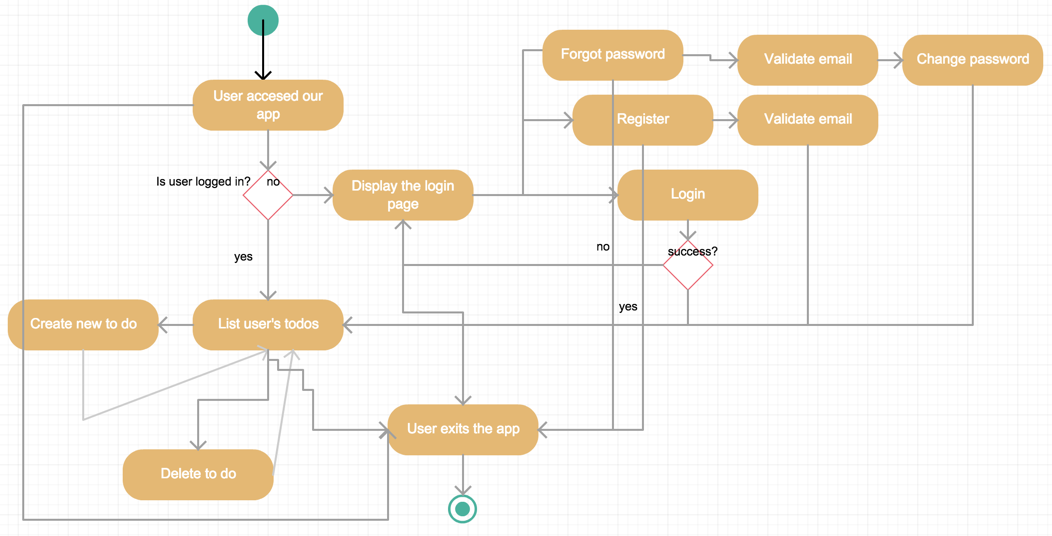 | ||
+ | ||
+A user accesses our to-do application. | ||
+- If the user is not logged in she will see our login page. | ||
+- If she already has an account, she can log in. | ||
+- If she has an account, but she forgot her password, she can recover her password. | ||
+- If she doesn't have an account, she can create one. | ||
+- Both "create an account" and "recover my password" will require email validation. A user can log in to our application only after she has confirmed her email address. | ||
+- If she is logged in, she will see her to-do list (this can be empty if she hasn't added any to-dos yet). | ||
+- A logged in user: | ||
+ - is able to see her tasks list | ||
+ - is able to mark a task from her list as completed | ||
+ - is able to search within her task list | ||
+ - is able to delete a task from her list | ||
+ - can logout. | ||
+ | ||
+- The user can exit the application at any time. | ||
+ | ||
+## 5) Create our mockups | ||
+Our mockups show what our app should look like. It's much faster to iterate on a mockup than it is to do so on working code. | ||
+ | ||
+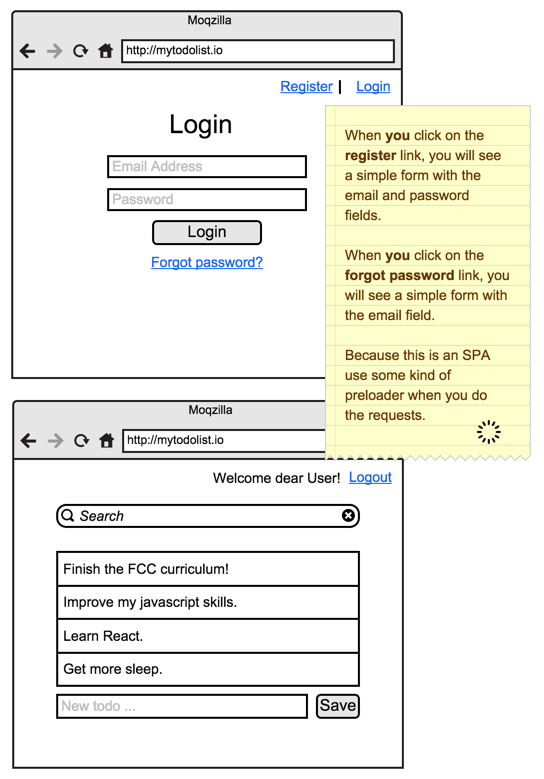 | ||
+ | ||
+## 6) Choose the right technologies for our project | ||
+Because this is a single page app, we'll rely heavily - or in this case exclusively - on JavaScript. Let's use one of the most popular JavaScript stacks: the MEAN stack. One big benefit of the MEAN stack is that all of its components are free and open-source. There are also tons of resources available for learning the MEAN stack, and for debugging it when you inevitably encounter errors. | ||
+ | ||
+You can have a [MEAN stack development environment](http://www.freecodecamp.com/challenges/get-set-for-our-back-end-development-projects) up and running in the cloud in less than an hour, for free. | ||
+ | ||
+Here are the components we'll use: | ||
+1. [MongoDB](http://mongodb.org/) for our database | ||
+2. [Node.js](http://nodejs.org/) and [Express.js](http://expressjs.com/) for implementing our API | ||
+3. [AngularJS](http://angularjs.org/), along with HTML and CSS (and Bootstrap) for our client-side application | ||
+4. [Mongoose](http://mongoosejs.com/) to connect our application to MongoDB | ||
+ | ||
+## 7) Design our database schema | ||
+It's worth the effort to design a database schema, even for our simple application. | ||
+ | ||
+We'll have two collections: our "Users" collection will house our user data, and our "ToDo" collection will house our tasks that need to be done. One user can have zero, one, or many tasks in her to-do list, so we will have a one-to-many (1:m) relationship between our two collections. | ||
+ | ||
+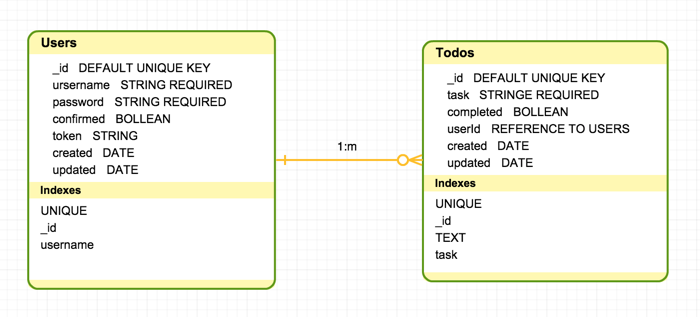 | ||
+ | ||
+## 8) Define our use cases | ||
+1. What happens to the to-dos related to a user that deletes her account? When the user deletes her account the to-dos related to that user should also be deleted. | ||
+2. No to-do can be added without being attached to a confirmed user. | ||
+3. A to-do can only be deleted by its owner. | ||
+4. No user can be added with an empty username or password. | ||
+5. No to-do can be added with an empty task. | ||
+ | ||
+Things to keep in mind: | ||
+1. Use the Mongoose middleware to remove dependent documents like to-dos when a user deletes her account. | ||
+2. Use Mongoose validation rules on models to prevent empty fields from being added to our database. | ||
+ | ||
+## 9) Design and test our API | ||
+I used a free product called Apiary [to document our API](http://docs.fcctodoapp.apiary.io/). | ||
+ | ||
+Here's the syntax I used to [create this simple BluePrint](https://jsapi.apiary.io/apis/fcctodoapp.apib). | ||
+ | ||
+I recommend you create an account and start playing with it. If you link your [GitHub](http://github.com/) account with Apiary, you can ensure your documentation always stays up to date. You'll also be able to test your data visually without the need for actually hitting your API endpoints. If you prefer to test your API from the command line, [here's an example of how to do this](http://docs.agendor.apiary.io/). | ||
+ | ||
+Later, once you've implemented your API with Node.js and Express.js, you'll just need to set your URL in Apiary. Then you can start testing your calls. Our current host url ([http://fcctodoapp.apiblueprint.org/](http://fcctodoapp.apiblueprint.org/)) will be replaced by your API's URL. | ||
+ | ||
+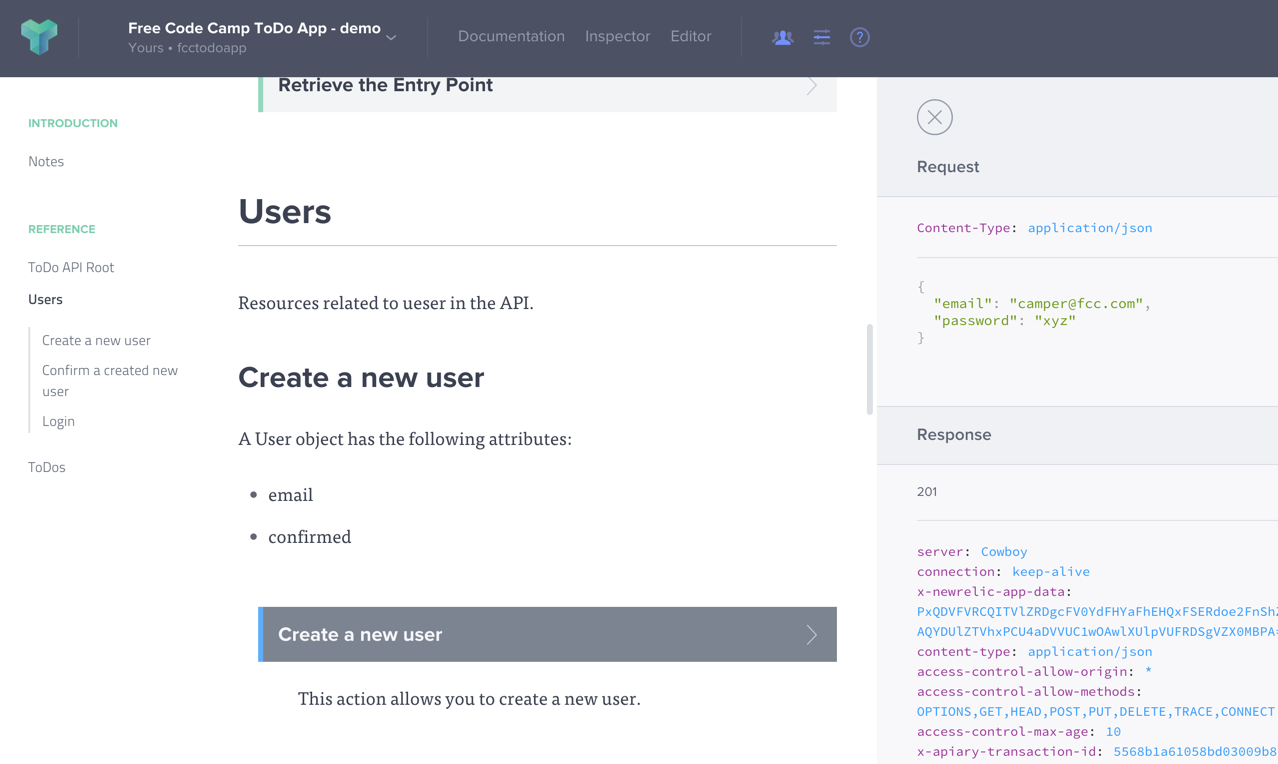 | ||
+ | ||
+## 10) Start writing code! | ||
+This is the fun part, and it will take up most of your project's time. If you need help with this, join us and learn to code. |
305
Algorithm-Missing-letters.md
@@ -1,151 +1,154 @@ | ||
-# Problem Explanation: | ||
-- You will create a program that will find the missing letter from a string and add it. If there is not missing letter it will return undefined. There is currently no test case for it missing more than one letter, but if anything recursion can be implemented or a second or more calls to the same function as needed. Also the letters are always provided in order so there is no need to sort them. | ||
- | ||
-## Hint: 1 | ||
-- You will need to convert from character to ASCII code using the two methods provided in the description. | ||
- | ||
-## Hint: 2 | ||
-- You will have to check for the difference in ASCII code as they are in order. Using a chart would be very helpful. | ||
- | ||
-## Hint: 3 | ||
-- You will need to figure out where to insert the letter and how to do it, along with handling the case that there is not missing letter as it needs an specific return value. | ||
- | ||
-## Spoiler Alert! | ||
-[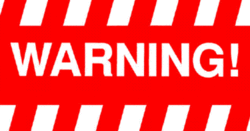](https://files.gitter.im/FreeCodeCamp/Wiki/nlOm/687474703a2f2f7777772e796f75726472756d2e636f6d2f796f75726472756d2f696d616765732f323030372f31302f31302f7265645f7761726e696e675f7369676e5f322e676966.gif) | ||
- | ||
-**Solution ahead!** | ||
- | ||
-## Code Solution: | ||
-####First solution: | ||
- | ||
-```js | ||
-function fearNotLetter(str) { | ||
- // Create our variables. | ||
- var firstCharacter = str.charCodeAt(0); | ||
- var valueToReturn = ''; | ||
- var secondCharacter = ''; | ||
- | ||
- // Function to find the missing letters | ||
- var addCharacters = function(a, b) { | ||
- while (a - 1 > b) { | ||
- b++; | ||
- valueToReturn += String.fromCharCode(b); | ||
- } | ||
- return valueToReturn; | ||
- }; | ||
- | ||
- // Check if letters are missing in between. | ||
- for (var index = 1; index < str.length; index++) { | ||
- secondCharacter = str.charCodeAt(index); | ||
- | ||
- // Check if the diference in code is greater than one. | ||
- if (secondCharacter - firstCharacter > 1) { | ||
- // Call function to missing letters | ||
- addCharacters(secondCharacter, firstCharacter); | ||
- } | ||
- | ||
- // Switch positions | ||
- firstCharacter = str.charCodeAt(index); | ||
- } | ||
- | ||
- // Check whether to return undefined or the missing letters. | ||
- if (valueToReturn === '') | ||
- return undefined; | ||
- else | ||
- return valueToReturn; | ||
-} | ||
-``` | ||
- | ||
-####Second solution: | ||
- | ||
-```js | ||
-function fearNotLetter(str) { | ||
- | ||
- for(var i = 0; i < str.length; i++) { | ||
- /* code of current character */ | ||
- var code = str.charCodeAt(i); | ||
- | ||
- /* if code of current character is not equal to first charcter + no of iteration | ||
- hence character has been escaped*/ | ||
- if ( code !== str.charCodeAt(0) + i) { | ||
- | ||
- /* if current character has escaped one character find previous char and return*/ | ||
- return String.fromCharCode(code-1); | ||
- } | ||
- } | ||
- return undefined; | ||
-} | ||
-``` | ||
- | ||
-####Third solution (Declarative) | ||
-```js | ||
-// Adding this solution for the sake of avoiding using 'for' and 'while' loops. | ||
-// See the explanation for reference as to why. It's worth the effort. | ||
- | ||
-function fearNotLetter(str) { | ||
- var compare = str.charCodeAt(0), missing; | ||
- | ||
- str.split('').map(function(letter,index){ | ||
- if (str.charCodeAt(index) == compare) { ++compare; } | ||
- else { missing = String.fromCharCode(compare); } | ||
- }); | ||
- | ||
- return missing; | ||
-} | ||
-``` | ||
- | ||
-####Fourth solution: | ||
-```js | ||
-function fearNotLetter(str) { | ||
- var strArr = str.split(''); | ||
- var missingChars = [], i = 0; | ||
- var nextChar = String.fromCharCode(strArr[i].charCodeAt(0)+1); | ||
- while (i<strArr.length - 1) { | ||
- if (nextChar !== strArr[i+1]){ | ||
- missingChars.push(nextChar); | ||
- nextChar = String.fromCharCode(nextChar.charCodeAt(0)+1); | ||
- } else { | ||
- i++; | ||
- nextChar = String.fromCharCode(strArr[i].charCodeAt(0)+1); | ||
- } | ||
- } | ||
- return missingChars.join('') === '' ? undefined : missingChars.join('') ; | ||
-} | ||
-``` | ||
- | ||
-####Fifth solution: | ||
-```js | ||
-function fearNotLetter(str) { | ||
- var allChars = ''; | ||
- var notChars = new RegExp('[^'+str+']','g'); | ||
- for (var i=0;allChars[allChars.length-1] !== str[str.length-1] ;i++) | ||
- allChars += String.fromCharCode(str[0].charCodeAt(0)+i); | ||
- return allChars.match(notChars) ? allChars.match(notChars).join('') : undefined; | ||
-} | ||
-``` | ||
-# Code Explanation: | ||
-####First and second solutions: | ||
-- Read comments in code. | ||
- | ||
-####Third solution (Declarative): | ||
-- First we define variables to store the character code for the first letter in the string, and to store whatever missing letters we may find. | ||
-- We turn the string to an array in order to map through it instead of using those nasty `for` and `whiles` (See [this article](http://www.sitepoint.com/quick-tip-stop-writing-loops-start-thinking-with-maps/) for refence as to why. And give it a serious try. Really... you'll thank me for it. | ||
-(If you're not sure how to `map`, you can review [Challenge: Iterate over arrays with map] ](http://www.freecodecamp.com/challenges/Challenge-iterate-over-arrays-with-map) and [MDN's reference](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map)). | ||
-- As we `map` through our letters' character codes, we go comparing with the one that should be in that position. | ||
-- If the current letter matches, we move the comparison variable to its next position so we can compare on the next cycle. | ||
-- if not, the missing letter will be assigned to the `missing` variable, which will be returned after the map is finished. | ||
- | ||
-####Fourth solution: | ||
-- Increase loop index only when you have found all the missing letters between current and next letter | ||
-- Every time you find a missing letter push it to `missingchars` | ||
- | ||
-####Fifth solution: | ||
-- Create a new String that consists all the letters in the range | ||
-- Create a Regular Expression for anything except `str` | ||
-- Use `match()` to strip off the `str` letters from your newly created String | ||
- | ||
- | ||
-# Credits: | ||
-If you found this page useful, you can give thanks by copying and pasting this on the main chat: **`thanks @Rafase282 @rohitnwn @sabahang @Hallaathrad for your help with Algorithm: Missing Letters`** | ||
- | ||
-> **NOTE:** Please add your username only if you have added any **relevant main contents** to the wiki page. (Please don't remove any existing usernames.) | ||
+# Problem Explanation: | ||
+- You will create a program that will find the missing letter from a string and add it. If there is not missing letter it will return undefined. There is currently no test case for it missing more than one letter, but if anything recursion can be implemented or a second or more calls to the same function as needed. Also the letters are always provided in order so there is no need to sort them. | ||
+ | ||
+## Hint: 1 | ||
+- You will need to convert from character to ASCII code using the two methods provided in the description. | ||
+ | ||
+## Hint: 2 | ||
+- You will have to check for the difference in ASCII code as they are in order. Using a chart would be very helpful. | ||
+ | ||
+## Hint: 3 | ||
+- You will need to figure out where to insert the letter and how to do it, along with handling the case that there is not missing letter as it needs an specific return value. | ||
+ | ||
+## Spoiler Alert! | ||
+[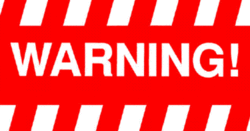](https://files.gitter.im/FreeCodeCamp/Wiki/nlOm/687474703a2f2f7777772e796f75726472756d2e636f6d2f796f75726472756d2f696d616765732f323030372f31302f31302f7265645f7761726e696e675f7369676e5f322e676966.gif) | ||
+ | ||
+**Solution ahead!** | ||
+ | ||
+## Code Solution: | ||
+### First solution: | ||
+ | ||
+```js | ||
+function fearNotLetter(str) { | ||
+ // Create our variables. | ||
+ var firstCharacter = str.charCodeAt(0); | ||
+ var valueToReturn = ''; | ||
+ var secondCharacter = ''; | ||
+ | ||
+ // Function to find the missing letters | ||
+ var addCharacters = function(a, b) { | ||
+ while (a - 1 > b) { | ||
+ b++; | ||
+ valueToReturn += String.fromCharCode(b); | ||
+ } | ||
+ return valueToReturn; | ||
+ }; | ||
+ | ||
+ // Check if letters are missing in between. | ||
+ for (var index = 1; index < str.length; index++) { | ||
+ secondCharacter = str.charCodeAt(index); | ||
+ | ||
+ // Check if the diference in code is greater than one. | ||
+ if (secondCharacter - firstCharacter > 1) { | ||
+ // Call function to missing letters | ||
+ addCharacters(secondCharacter, firstCharacter); | ||
+ } | ||
+ | ||
+ // Switch positions | ||
+ firstCharacter = str.charCodeAt(index); | ||
+ } | ||
+ | ||
+ // Check whether to return undefined or the missing letters. | ||
+ if (valueToReturn === '') | ||
+ return undefined; | ||
+ else | ||
+ return valueToReturn; | ||
+} | ||
+``` | ||
+ | ||
+### Second solution: | ||
+ | ||
+```js | ||
+function fearNotLetter(str) { | ||
+ | ||
+ for(var i = 0; i < str.length; i++) { | ||
+ /* code of current character */ | ||
+ var code = str.charCodeAt(i); | ||
+ | ||
+ /* if code of current character is not equal to first charcter + no of iteration | ||
+ hence character has been escaped*/ | ||
+ if ( code !== str.charCodeAt(0) + i) { | ||
+ | ||
+ /* if current character has escaped one character find previous char and return*/ | ||
+ return String.fromCharCode(code-1); | ||
+ } | ||
+ } | ||
+ return undefined; | ||
+} | ||
+``` | ||
+ | ||
+### Third solution (Declarative) | ||
+ | ||
+```js | ||
+// Adding this solution for the sake of avoiding using 'for' and 'while' loops. | ||
+// See the explanation for reference as to why. It's worth the effort. | ||
+ | ||
+function fearNotLetter(str) { | ||
+ var compare = str.charCodeAt(0), missing; | ||
+ | ||
+ str.split('').map(function(letter,index){ | ||
+ if (str.charCodeAt(index) == compare) { ++compare; } | ||
+ else { missing = String.fromCharCode(compare); } | ||
+ }); | ||
+ | ||
+ return missing; | ||
+} | ||
+``` | ||
+ | ||
+### Fourth solution: | ||
+ | ||
+```js | ||
+function fearNotLetter(str) { | ||
+ var strArr = str.split(''); | ||
+ var missingChars = [], i = 0; | ||
+ var nextChar = String.fromCharCode(strArr[i].charCodeAt(0)+1); | ||
+ while (i<strArr.length - 1) { | ||
+ if (nextChar !== strArr[i+1]){ | ||
+ missingChars.push(nextChar); | ||
+ nextChar = String.fromCharCode(nextChar.charCodeAt(0)+1); | ||
+ } else { | ||
+ i++; | ||
+ nextChar = String.fromCharCode(strArr[i].charCodeAt(0)+1); | ||
+ } | ||
+ } | ||
+ return missingChars.join('') === '' ? undefined : missingChars.join('') ; | ||
+} | ||
+``` | ||
+ | ||
+### Fifth solution: | ||
+ | ||
+```js | ||
+function fearNotLetter(str) { | ||
+ var allChars = ''; | ||
+ var notChars = new RegExp('[^'+str+']','g'); | ||
+ for (var i=0;allChars[allChars.length-1] !== str[str.length-1] ;i++) | ||
+ allChars += String.fromCharCode(str[0].charCodeAt(0)+i); | ||
+ return allChars.match(notChars) ? allChars.match(notChars).join('') : undefined; | ||
+} | ||
+``` | ||
+ | ||
+# Code Explanation: | ||
+## First and second solutions: | ||
+- Read comments in code. | ||
+ | ||
+## Third solution (Declarative): | ||
+- First we define variables to store the character code for the first letter in the string, and to store whatever missing letters we may find. | ||
+- We turn the string to an array in order to map through it instead of using those nasty `for` and `whiles` (See [this article](http://www.sitepoint.com/quick-tip-stop-writing-loops-start-thinking-with-maps/) for refence as to why. And give it a serious try. Really... you'll thank me for it. | ||
+- (If you're not sure how to `map`, you can review [Challenge: Iterate over arrays with map] ](http://www.freecodecamp.com/challenges/iterate-over-arrays-with-map) and [MDN's reference](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map)). | ||
+- As we `map` through our letters' character codes, we go comparing with the one that should be in that position. | ||
+- If the current letter matches, we move the comparison variable to its next position so we can compare on the next cycle. | ||
+- if not, the missing letter will be assigned to the `missing` variable, which will be returned after the map is finished. | ||
+ | ||
+## Fourth solution: | ||
+- Increase loop index only when you have found all the missing letters between current and next letter | ||
+- Every time you find a missing letter push it to `missingchars` | ||
+ | ||
+## Fifth solution: | ||
+- Create a new String that consists all the letters in the range | ||
+- Create a Regular Expression for anything except `str` | ||
+- Use `match()` to strip off the `str` letters from your newly created String | ||
+ | ||
+# Credits: | ||
+If you found this page useful, you can give thanks by copying and pasting this on the main chat: **`thanks @Rafase282 @rohitnwn @sabahang @Hallaathrad for your help with Algorithm: Missing Letters`** | ||
+ | ||
+> **NOTE:** Please add your username only if you have added any **relevant main contents** to the wiki page. (Please don't remove any existing usernames.) |
80
Algorithm-Style-Guide.md
@@ -1,40 +1,40 @@ | ||
-_work in progress_ | ||
- | ||
-# Algorithm Style Guide | ||
-Writing Algorithm challenges is a great way to exercise your own problem solving and testing abilities. It is a simple three step process. | ||
-1. Fill out the [generator](http://www.freecodecamp.com/algorithm-challenge-generator) form and test your challenge. | ||
-2. Once you have confirmed a working Algorithm challenge in the generator, copy and paste the fields into the [JSON generator.](http://www.freecodecamp.com/algorithm-json-generator) | ||
-3. Copy the JSON, fork the freecodecamp repository, and submit a pull request with your addition to the challenge .json file. | ||
- | ||
-## Name | ||
-Name your challenge | ||
- | ||
-## Difficulty | ||
-Attempt to rate difficulty compared against existing Algorithm challenges. | ||
- | ||
-_TODO: add basic quantifiers for each difficulty level_ | ||
- | ||
-## Description | ||
-Separate paragraphs with a line break. Only the first paragraph is visible prior to a user clicking the "More information" button. | ||
- | ||
-All necessary information must be included in the first paragraph. Write this first paragraph as succinct as possible. Subsequent paragraphs should offer hints or details if needed. | ||
- | ||
-If your subject matter warrants deeper understanding, you may link to Wikipedia. | ||
- | ||
-## Challenge Seed | ||
-This is important. | ||
- | ||
-## ~~Challenge Entry Point~~ (deprecated) | ||
-_Leave this field blank. It will soon be removed from the generator._ | ||
- | ||
-## Tests | ||
-These tests are what bring your challenge to life. Without them, we cannot confirm the accuracy of a user's submitted answer. Choose your tests wisely. | ||
- | ||
-Algorithm tests are written using the [Chai.js](http://chaijs.com/) assertion library. Please use the `should` and `expect` syntax for end user readability. As an example of what not do to, many of the original Algorithm challenges are written with `assert` syntax and many of the test cases are difficult to read. | ||
- | ||
-If your Algorithm question has a lot of edge cases, you will need to write many tests for full coverage. If you find yourself writing more tests than you desire, you may consider simplifying the requirements of your Algorithm challenge. For difficulty level 1 through 3, you will generally only need 2 to 4 tests. | ||
- | ||
-# Credits: | ||
-If you found this page useful, you can give thanks by copying and pasting this on the main chat: **`Thanks @brandenbyers @Rafase282 for your help with the Algorithm Style Guide`** | ||
- | ||
-> **NOTE:** Please add your username only if you have added any **relevant main contents** to the wiki page. (Please don't remove any existing usernames.) | ||
+_work in progress_ | ||
+ | ||
+# Algorithm Style Guide | ||
+Writing Algorithm challenges is a great way to exercise your own problem solving and testing abilities. It is a simple three step process. | ||
+1. Fill out the [generator](http://www.freecodecamp.com/algorithm-challenge-generator) form and test your challenge. | ||
+2. Once you have confirmed a working Algorithm challenge in the generator, copy and paste the fields into the [JSON generator.](http://www.freecodecamp.com/algorithm-json-generator) | ||
+3. Copy the JSON, fork the freecodecamp repository, and submit a pull request with your addition to the challenge .json file. | ||
+ | ||
+## Name | ||
+Name your challenge | ||
+ | ||
+## Difficulty | ||
+Attempt to rate difficulty compared against existing Algorithm challenges. | ||
+ | ||
+_TODO: add basic quantifiers for each difficulty level_ | ||
+ | ||
+## Description | ||
+Separate paragraphs with a line break. Only the first paragraph is visible prior to a user clicking the "More information" button. | ||
+ | ||
+All necessary information must be included in the first paragraph. Write this first paragraph as succinct as possible. Subsequent paragraphs should offer hints or details if needed. | ||
+ | ||
+If your subject matter warrants deeper understanding, you may link to Wikipedia. | ||
+ | ||
+## Challenge Seed | ||
+This is important. | ||
+ | ||
+## ~~Challenge Entry Point~~ (deprecated) | ||
+_Leave this field blank. It will soon be removed from the generator._ | ||
+ | ||
+## Tests | ||
+These tests are what bring your challenge to life. Without them, we cannot confirm the accuracy of a user's submitted answer. Choose your tests wisely. | ||
+ | ||
+Algorithm tests are written using the [Chai.js](http://chaijs.com/) assertion library. Please use the `should` and `expect` syntax for end user readability. As an example of what not do to, many of the original Algorithm challenges are written with `assert` syntax and many of the test cases are difficult to read. | ||
+ | ||
+If your Algorithm question has a lot of edge cases, you will need to write many tests for full coverage. If you find yourself writing more tests than you desire, you may consider simplifying the requirements of your Algorithm challenge. For difficulty level 1 through 3, you will generally only need 2 to 4 tests. | ||
+ | ||
+# Credits: | ||
+If you found this page useful, you can give thanks by copying and pasting this on the main chat: **`Thanks @brandenbyers @Rafase282 for your help with the Algorithm Style Guide`** | ||
+ | ||
+> **NOTE:** Please add your username only if you have added any **relevant main contents** to the wiki page. (Please don't remove any existing usernames.) |
77
Back-End-Project-Resources.md
@@ -1,36 +1,41 @@ | ||
-The curriculum lead-up to the first Back End Project is not very comprehensive. Here are a number of common resources which other campers have found helpful. | ||
- | ||
-### Getting Started with Back End Projects | ||
-* https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Intro-to-Yeoman-Angular-Fullstack-Back End Projects - Lots of helpful tips and tricks for the Yeoman Angular Fullstack setup | ||
-* https://github.com/DaftMonk/generator-angular-fullstack#generators - Generator used by Yeoman, you can find syntax and what files it creates | ||
- | ||
-### APIs | ||
-* API for Charting the stock market: https://www.quandl.com/help/api | ||
- | ||
-### MEAN Stack Tutorials & Videos | ||
-* 5 Part Series on setting up a MEAN stack | ||
-https://www.youtube.com/watch?v=kHV7gOHvNdk | ||
- | ||
-* A MEAN tutorial that creates a simple Twitter clone | ||
-https://channel9.msdn.com/Series/MEAN-Stack-Jump-Start | ||
- | ||
-* Clementine is a stripped down MEAN stack, great for learning the fundamentals. | ||
-https://johnstonbl01.github.io/clementinejs/tutorials/tutorial-beginner.html | ||
- | ||
-* Authentication with Passport for the MEAN stack: | ||
-https://vickev.com/#!/article/authentication-in-single-page-applications-node-js-passportjs-angularjs | ||
- | ||
-* An amazing list of resources for learning the MEAN stack: | ||
-https://github.com/ericdouglas/MEAN-Learning | ||
- | ||
-### Scotch IO Tutorials | ||
-* https://scotch.io/tutorials/setting-up-a-mean-stack-single-page-application | ||
-* https://scotch.io/tutorials/node-and-angular-to-do-app-application-organization-and-structure | ||
- | ||
-### Node/Express | ||
-* [Online Debugging for Node.js/Express](http://stackoverflow.com/a/16512303/1420506) | ||
- | ||
-### Cloud 9 Tricks | ||
-##### Speed up browser reloads | ||
-1. Open gruntfile.js and edit both instances of `livereload: true` to `livereload: false`. | ||
-2. Open server/config/express.js and comment out the line `app.use(require('connect-livereload')());` | ||
+The curriculum lead-up to the first Back End Project is not very comprehensive. Here are a number of common resources which other campers have found helpful. | ||
+ | ||
+# Getting Started with Back End Projects | ||
+- [https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Intro-to-Yeoman-Angular-Fullstack-Back-End-Projects](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Intro-to-Yeoman-Angular-Fullstack-Back-End-Projects) - Lots of helpful tips and tricks for the Yeoman Angular Fullstack setup | ||
+- [https://github.com/DaftMonk/generator-angular-fullstack#generators](https://github.com/DaftMonk/generator-angular-fullstack#generators) - Generator used by Yeoman, you can find syntax and what files it creates | ||
+ | ||
+# APIs | ||
+- API for Charting the stock market: [https://www.quandl.com/help/api](https://www.quandl.com/help/api) | ||
+ | ||
+# MEAN Stack Tutorials & Videos | ||
+- 5 Part Series on setting up a MEAN stack | ||
+ | ||
+ [https://www.youtube.com/watch?v=kHV7gOHvNdk](https://www.youtube.com/watch?v=kHV7gOHvNdk) | ||
+ | ||
+- A MEAN tutorial that creates a simple Twitter clone | ||
+ | ||
+ [https://channel9.msdn.com/Series/MEAN-Stack-Jump-Start](https://channel9.msdn.com/Series/MEAN-Stack-Jump-Start) | ||
+ | ||
+- Clementine is a stripped down MEAN stack, great for learning the fundamentals. | ||
+ | ||
+ [https://johnstonbl01.github.io/clementinejs/tutorials/tutorial-beginner.html](https://johnstonbl01.github.io/clementinejs/tutorials/tutorial-beginner.html) | ||
+ | ||
+- Authentication with Passport for the MEAN stack: | ||
+ | ||
+ [https://vickev.com/#!/article/authentication-in-single-page-applications-node-js-passportjs-angularjs](https://vickev.com/#!/article/authentication-in-single-page-applications-node-js-passportjs-angularjs) | ||
+ | ||
+- An amazing list of resources for learning the MEAN stack: | ||
+ | ||
+ [https://github.com/ericdouglas/MEAN-Learning](https://github.com/ericdouglas/MEAN-Learning) | ||
+ | ||
+# Scotch IO Tutorials | ||
+- [https://scotch.io/tutorials/setting-up-a-mean-stack-single-page-application](https://scotch.io/tutorials/setting-up-a-mean-stack-single-page-application) | ||
+- [https://scotch.io/tutorials/node-and-angular-to-do-app-application-organization-and-structure](https://scotch.io/tutorials/node-and-angular-to-do-app-application-organization-and-structure) | ||
+ | ||
+# Node/Express | ||
+- [Online Debugging for Node.js/Express](http://stackoverflow.com/a/16512303/1420506) | ||
+ | ||
+# Cloud 9 Tricks | ||
+## Speed up browser reloads | ||
+1. Open gruntfile.js and edit both instances of `livereload: true` to `livereload: false`. | ||
+2. Open server/config/express.js and comment out the line `app.use(require('connect-livereload')());` |
10
Checkpoint-Counting-Cards.md
6
Checkpoint-Golf-Code.md
6
Checkpoint-Profile-Lookup.md
8
Checkpoint-Record-Collection.md
2
Checkpoint-Shopping-List.md
12
Checkpoint-Stand-In-Line.md
6
Checkpoint-Word-Blanks.md
14
Contributions-Guide---with-Typo-Demo.md
20
Frontend-file-structure.md
@@ -1,11 +1,9 @@ | ||
-First things first: All your user-facing files and angular files are in **/client/app/** | ||
- | ||
-1. **app.js**: defines your app and includes some basic app-wide functions, you probably don’t really need to mess with it unless you’re trying to add more dependencies to your app. We’re not gonna worry about that right now. | ||
-2. **app.css**: an app-wide stylesheet, you can put styles here if you want but I’d recommend you put them in **main/main.css**, as these styles are also app-wide. | ||
-3. **main/**: this folder contains what the user sees first when they load up your site. **main.html** is the page template, **main.js** routes the user to **main.html** when the user goes to the top level directory of your website—that is, <a href="#">http://yourapp.wherever.itis/</a> with no <a href="#">/other/url/hierarchy</a>. You’ll also learn soon that you can define your app’s <a href="#">/url/heirarchy/fairly/arbitrarily</a>. You won’t really need to edit **main.js** or **main.controller.spec.js**, so let's not worry about those right now. If you look through the **main.html** file you’ll see it uses *ng-repeat* to show *things* in *awesomeThings*. Where does it get *awesomeThings*? | ||
-4. **main/main.controller.js**: all of the javascript functions you want to use to interact directly with the user go here! You’ll put functions here to interact with your API, refresh views for your user, etc. Here, *awesomeThings* are pulled from your database and added to the local scope so your HTML view can display them! How cool! We’ll get to adding custom objects to your database in a minute. | ||
- | ||
-Great! Now you know how to interact with the user! But what if you want your app to have another page that does something else? Maybe **main.html** shows the home page, but you want a page that shows a form to add a poll? maybe <a href="#">http://yourapp.wherever.itis/newpage</a>? This is where the yeoman generator comes in handy. | ||
- | ||
-[PREVIOUS](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Guide-to-Back End Projects-Table-of-Contents/) | ||
-[NEXT](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Creating-a-new-route) | ||
+First things first: All your user-facing files and angular files are in **/client/app/** | ||
+1. **app.js**: defines your app and includes some basic app-wide functions, you probably don't really need to mess with it unless you're trying to add more dependencies to your app. We're not gonna worry about that right now. | ||
+2. **app.css**: an app-wide stylesheet, you can put styles here if you want but I'd recommend you put them in **main/main.css**, as these styles are also app-wide. | ||
+3. **main/**: this folder contains what the user sees first when they load up your site. **main.html** is the page template, **main.js** routes the user to **main.html** when the user goes to the top level directory of your website--that is, [http://yourapp.wherever.itis/](#) with no [/other/url/hierarchy](#). You'll also learn soon that you can define your app's [/url/heirarchy/fairly/arbitrarily](#). You won't really need to edit **main.js** or **main.controller.spec.js**, so let's not worry about those right now. If you look through the **main.html** file you'll see it uses _ng-repeat_ to show _things_ in _awesomeThings_. Where does it get _awesomeThings_? | ||
+4. **main/main.controller.js**: all of the javascript functions you want to use to interact directly with the user go here! You'll put functions here to interact with your API, refresh views for your user, etc. Here, _awesomeThings_ are pulled from your database and added to the local scope so your HTML view can display them! How cool! We'll get to adding custom objects to your database in a minute. | ||
+ | ||
+Great! Now you know how to interact with the user! But what if you want your app to have another page that does something else? Maybe **main.html** shows the home page, but you want a page that shows a form to add a poll? maybe [http://yourapp.wherever.itis/newpage](#)? This is where the yeoman generator comes in handy. | ||
+ | ||
+[PREVIOUS](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Guide-to-Back-End-Projects-Table-of-Contents) [NEXT](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Creating-a-new-route) |
64
Guide-to-Back-End-Projects-Table-of-Contents.md
@@ -1,31 +1,33 @@ | ||
-###Table of contents | ||
-* Part 1: Frontend | ||
- - [Frontend file structure](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Frontend-file-structure) | ||
- - [Creating a new route](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Creating-a-new-route) | ||
- - [Creating a new directive](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Creating-a-new-directive) | ||
- - [Grunt](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Grunt) | ||
-* Part 2: Backend | ||
- - [Backend file structure](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Backend-file-structure) | ||
- - [Creating a new API endpoint](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Creating-a-new-API-endpoint) | ||
- - [Fixing exports.update](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Fixing-exports.update) | ||
-* Part 3: Interfacing Between Frontend & Backend | ||
- - [Accessing the database from your frontend](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Accessing-the-database-from-your-frontend) | ||
- - [Seed Data](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Seed-data) | ||
- - [Quick tip: keep data in sync](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Quick-tip-keep-data-in-sync) | ||
-* Part 4: Dynamic URLs using $routeParams, more useful APIs | ||
- - [Dynamic URLS using $routeParams](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Dynamic-URLs-using-$routeParams) | ||
- - [More Useful APIs](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/More-useful-APIs) | ||
-* Part 5: Auth, isLoggedInAsync() | ||
- - [Get info about the current user](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Get-info-about-the-current-user) | ||
- - [Restrict a page to authenticated users](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Restrict-a-page-to-authenticated-users) | ||
- - [isLoggedInAsync()](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/isLoggedInAsync()) | ||
-* [Bonus: SocketIO](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Bonus-SocketIO) | ||
-* [Epilogue](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Epilogue) | ||
- | ||
-###Legend | ||
-**/bolded/names/with.extensions** are directories and files in the project file structure | ||
-<a href="#">highlighted.items/are/hypothetical</a> URLs that allow access to different pages in your app | ||
-*italicizedItems* are function and object names within your code | ||
- | ||
-[PREVIOUS](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Intro-to-Yeoman-Angular-Fullstack-Back End Projects) | ||
-[NEXT](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Frontend-file-structure) | ||
+# Table of contents | ||
+- Part 1: Frontend | ||
+ - [Frontend file structure](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Frontend-file-structure) | ||
+ - [Creating a new route](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Creating-a-new-route) | ||
+ - [Creating a new directive](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Creating-a-new-directive) | ||
+ - [Grunt](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Grunt) | ||
+ | ||
+- Part 2: Backend | ||
+ - [Backend file structure](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Backend-file-structure) | ||
+ - [Creating a new API endpoint](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Creating-a-new-API-endpoint) | ||
+ - [Fixing exports.update](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Fixing-exports.update) | ||
+ | ||
+- Part 3: Interfacing Between Frontend & Backend | ||
+ - [Accessing the database from your frontend](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Accessing-the-database-from-your-frontend) | ||
+ - [Seed Data](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Seed-data) | ||
+ - [Quick tip: keep data in sync](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Quick-tip-keep-data-in-sync) | ||
+ | ||
+- Part 4: Dynamic URLs using $routeParams, more useful APIs | ||
+ - [Dynamic URLS using $routeParams](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Dynamic-URLs-using-$routeParams) | ||
+ - [More Useful APIs](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/More-useful-APIs) | ||
+ | ||
+- Part 5: Auth, isLoggedInAsync() | ||
+ - [Get info about the current user](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Get-info-about-the-current-user) | ||
+ - [Restrict a page to authenticated users](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Restrict-a-page-to-authenticated-users) | ||
+ - [isLoggedInAsync()](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/isLoggedInAsync()) | ||
+ | ||
+- [Bonus: SocketIO](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Bonus-SocketIO) | ||
+- [Epilogue](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Epilogue) | ||
+ | ||
+# Legend | ||
+**/bolded/names/with.extensions** are directories and files in the project file structure<br>[highlighted.items/are/hypothetical](#) URLs that allow access to different pages in your app<br>_italicizedItems_ are function and object names within your code | ||
+ | ||
+[PREVIOUS](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Intro-to-Yeoman-Angular-Fullstack-Back-End-Projects) [NEXT](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Frontend-file-structure) |
217
How-to-get-the-MEAN-stack-running-locally-on-OSX.md
@@ -1,97 +1,120 @@ | ||
-## Requirements | ||
-To install MongoDB, you should have Mac OS X 10.6 (Snow Leopard) or above. To find out which version of OS X you own, click the icon in the top left corner of your screen and select `About This Mac`. | ||
- | ||
-:warning: **WARNING:** do a Time Machine backup before carrying out any of the following steps! | ||
- | ||
-## Step 1: installing MongoDB | ||
-The easiest way to install MongoDB on OS X is using [HomeBrew](http://brew.sh/). If you haven't used HomeBrew before, simply execute the following command in a Terminal window: | ||
-```sh | ||
-ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)" | ||
-``` | ||
-After HomeBrew is successfully installed, follow up with this command: | ||
-```sh | ||
-brew update && brew install mongodb | ||
-``` | ||
-HomeBrew will automatically install all the dependencies for you. | ||
- | ||
-## Step 2: installing Node.js | ||
-Again, we'll let HomeBrew do the heavy lifting: | ||
-```sh | ||
-brew install node | ||
-``` | ||
-The npm executable is already included in the Node.js package. | ||
- | ||
-Before continuing, let's make sure Node.js modules can be found by others (:warning: **CAUTION**: it's best to copy and paste these commands, as you'd lose the original contents of your `.bashrc` file if you typed `>` in place of `>>`): | ||
-```sh | ||
-echo 'export NODE_PATH="./node_modules:/usr/local/lib/node_modules"' >> ~/.bashrc && source ~/.bashrc | ||
-``` | ||
-To check if the configuration is in effect, execute: | ||
-```sh | ||
-echo $NODE_PATH | ||
-``` | ||
-You should see `./node_modules:/usr/local/lib/node_modules` printed out below your command. | ||
- | ||
-If you use a different shell than Bash, simply replace `~/.bashrc` with your shell configuration file. | ||
- | ||
-## Step 3: installing fullstack tools | ||
-```sh | ||
-npm install -g express yo grunt grunt-cli generator-angular-fullstack bower | ||
-``` | ||
- | ||
-## Step 4: generating a fullstack app | ||
-Make a directory for your Back End Project projects. Assuming your desktop is your de facto workspace: | ||
-```sh | ||
-mkdir ~/Desktop/Back End Projects && cd ~/Desktop/Back End Projects | ||
-``` | ||
-Now that all the preparations are in place, it's time for the main event: | ||
-```sh | ||
-yo angular-fullstack | ||
-``` | ||
-Answer the questions according to checklist items #13-23 of [Challenge: Get Set for Back End Projects](http://www.freecodecamp.com/challenges/Challenge-get-set-for-Back End Projects). Consult #24-27 if you run into errors. This will download ~350MB worth of files into your current directory. | ||
- | ||
-Before going any further, we need to fix a [known issue](https://github.com/clnhll/guidetobasejumps#fixing-exportsupdate) in some generated files: | ||
-```sh | ||
-echo "sed -i '' -e 's/_.merge/_.extend/' server/api/*/*.controller.js" > \ | ||
-fix-exports-update.sh && chmod +x fix-exports-update.sh && \ | ||
-./fix-exports-update.sh | ||
-``` | ||
-You need to run `./fix-exports-update.sh` every time you generate a new API endpoint (until they fix this upstream, that is). | ||
- | ||
-## Step 5: initialising local Git repository | ||
-Tell Git not to track your database: | ||
-```sh | ||
-echo "data" >> .gitignore | ||
-``` | ||
- | ||
-Turn the directory in which your application is located into a Git repository by running the following commands: | ||
-```sh | ||
-git init && git add . && git commit -am 'initial commit' | ||
-``` | ||
- | ||
-## Step 6: starting MongoDB | ||
-To start MongoDB for the first time in your app's directory, run the following commands in your terminal: | ||
-```sh | ||
-mkdir data && echo 'mongod --config /usr/local/etc/mongod.conf --dbpath=data --rest "$@" --httpinterface' > mongod.sh && chmod a+x mongod.sh && ./mongod.sh | ||
-``` | ||
-From this point on you can simply start MongoDB by executing `./mongod.sh`. A few things to note: | ||
-* The `.conf` file directs `mongod` to write messages to a log file instead of stdout. To view the log, run the following in a separate Terminal tab: `less /usr/local/var/log/mongodb/mongo.log`. | ||
-* Since we're not on Cloud9, we don't need the `--nojournal` option. Journaling lets you recover the database in case of a `mongod` crash. | ||
-* You have to make a clean database for each project. If you copied the `data` directory over from an earlier project, `mongod` will fail to start. If that's the case, just `rm -rf data && mkdir data && ./mongod.sh`. | ||
- | ||
-## Step 7: starting Grunt | ||
-Open a new Terminal tab by pressing `⌘T`, and run the following command: | ||
-```sh | ||
-grunt serve | ||
-``` | ||
-Grunt should automatically open your new Angular site's index page as soon as it finishes starting up. | ||
- | ||
-Now you should be able to follow the rest of the Challenge instructions to push to GitHub and Heroku. Just ignore the part about SSH key (#33-36) and replace `~/workspace` with your app directory's path. | ||
- | ||
-*** | ||
- | ||
-##### Footnote | ||
-The above steps were tested under the following configuration: | ||
-* OS X 10.10.5 | ||
-* zsh 5.0.8 (x86_64-apple-darwin14.3.0) | ||
-* node v0.12.7 | ||
-* npm 2.11.3 | ||
+# Requirements | ||
+To install MongoDB, you should have Mac OS X 10.6 (Snow Leopard) or above. To find out which version of OS X you own, click the icon in the top left corner of your screen and select `About This Mac`. | ||
+ | ||
+:warning: **WARNING:** do a Time Machine backup before carrying out any of the following steps! | ||
+ | ||
+# Step 1: installing MongoDB | ||
+The easiest way to install MongoDB on OS X is using [HomeBrew](http://brew.sh/). If you haven't used HomeBrew before, simply execute the following command in a Terminal window: | ||
+ | ||
+```sh | ||
+ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)" | ||
+``` | ||
+ | ||
+After HomeBrew is successfully installed, follow up with this command: | ||
+ | ||
+```sh | ||
+brew update && brew install mongodb | ||
+``` | ||
+ | ||
+HomeBrew will automatically install all the dependencies for you. | ||
+ | ||
+# Step 2: installing Node.js | ||
+Again, we'll let HomeBrew do the heavy lifting: | ||
+ | ||
+```sh | ||
+brew install node | ||
+``` | ||
+ | ||
+The npm executable is already included in the Node.js package. | ||
+ | ||
+Before continuing, let's make sure Node.js modules can be found by others (:warning: **CAUTION**: it's best to copy and paste these commands, as you'd lose the original contents of your `.bashrc` file if you typed `>` in place of `>>`): | ||
+ | ||
+```sh | ||
+echo 'export NODE_PATH="./node_modules:/usr/local/lib/node_modules"' >> ~/.bashrc && source ~/.bashrc | ||
+``` | ||
+ | ||
+To check if the configuration is in effect, execute: | ||
+ | ||
+```sh | ||
+echo $NODE_PATH | ||
+``` | ||
+ | ||
+You should see `./node_modules:/usr/local/lib/node_modules` printed out below your command. | ||
+ | ||
+If you use a different shell than Bash, simply replace `~/.bashrc` with your shell configuration file. | ||
+ | ||
+# Step 3: installing fullstack tools | ||
+ | ||
+```sh | ||
+npm install -g express yo grunt grunt-cli generator-angular-fullstack bower | ||
+``` | ||
+ | ||
+# Step 4: generating a fullstack app | ||
+Make a directory for your Back End Project projects. Assuming your desktop is your de facto workspace: | ||
+ | ||
+```sh | ||
+mkdir ~/Desktop/Back End Projects && cd ~/Desktop/Back End Projects | ||
+``` | ||
+ | ||
+Now that all the preparations are in place, it's time for the main event: | ||
+ | ||
+```sh | ||
+yo angular-fullstack | ||
+``` | ||
+ | ||
+Answer the questions according to checklist items #13-23 of [Challenge: Get Set for Back End Projects](http://www.freecodecamp.com/challenges/get-set-for-our-back-end-development-projects). Consult #24-27 if you run into errors. This will download ~350MB worth of files into your current directory. | ||
+ | ||
+Before going any further, we need to fix a [known issue](https://github.com/clnhll/guidetobasejumps#fixing-exportsupdate) in some generated files: | ||
+ | ||
+```sh | ||
+echo "sed -i '' -e 's/_.merge/_.extend/' server/api/*/*.controller.js" > \ | ||
+fix-exports-update.sh && chmod +x fix-exports-update.sh && \ | ||
+./fix-exports-update.sh | ||
+``` | ||
+ | ||
+You need to run `./fix-exports-update.sh` every time you generate a new API endpoint (until they fix this upstream, that is). | ||
+ | ||
+# Step 5: initialising local Git repository | ||
+Tell Git not to track your database: | ||
+ | ||
+```sh | ||
+echo "data" >> .gitignore | ||
+``` | ||
+ | ||
+Turn the directory in which your application is located into a Git repository by running the following commands: | ||
+ | ||
+```sh | ||
+git init && git add . && git commit -am 'initial commit' | ||
+``` | ||
+ | ||
+# Step 6: starting MongoDB | ||
+To start MongoDB for the first time in your app's directory, run the following commands in your terminal: | ||
+ | ||
+```sh | ||
+mkdir data && echo 'mongod --config /usr/local/etc/mongod.conf --dbpath=data --rest "$@" --httpinterface' > mongod.sh && chmod a+x mongod.sh && ./mongod.sh | ||
+``` | ||
+ | ||
+From this point on you can simply start MongoDB by executing `./mongod.sh`. A few things to note: | ||
+- The `.conf` file directs `mongod` to write messages to a log file instead of stdout. To view the log, run the following in a separate Terminal tab: `less /usr/local/var/log/mongodb/mongo.log`. | ||
+- Since we're not on Cloud9, we don't need the `--nojournal` option. Journaling lets you recover the database in case of a `mongod` crash. | ||
+- You have to make a clean database for each project. If you copied the `data` directory over from an earlier project, `mongod` will fail to start. If that's the case, just `rm -rf data && mkdir data && ./mongod.sh`. | ||
+ | ||
+# Step 7: starting Grunt | ||
+Open a new Terminal tab by pressing `⌘T`, and run the following command: | ||
+ | ||
+```sh | ||
+grunt serve | ||
+``` | ||
+ | ||
+Grunt should automatically open your new Angular site's index page as soon as it finishes starting up. | ||
+ | ||
+Now you should be able to follow the rest of the Challenge instructions to push to GitHub and Heroku. Just ignore the part about SSH key (#33-36) and replace `~/workspace` with your app directory's path. | ||
+ | ||
+-------------------------------------------------------------------------------- | ||
+ | ||
+## Footnote | ||
+The above steps were tested under the following configuration: | ||
+- OS X 10.10.5 | ||
+- zsh 5.0.8 (x86_64-apple-darwin14.3.0) | ||
+- node v0.12.7 | ||
+- npm 2.11.3 |
18
Intro-to-Yeoman-Angular-Fullstack-Back-End-Projects.md
@@ -1,9 +1,9 @@ | ||
-> Hey FreeCodeCampers! This guide is here to help you navigate creating your first Back End Project. When I encountered the first Back End Project, I had no idea what was going on and spent weeks learning all of these things myself. Everything here is stuff I wish I had known coming into the Back End Projects. Oh and by the way, if you have a question that isn't answered by this guide, that's an issue, and you should report it as an issue to this repository! —[@clnhll](http://twitter.com/clnhll) | ||
- | ||
-Yeoman is a tool that allows you to generate barebones apps based on different software stacks using “generator” packages made by developers who want to make your life easier. These packages streamline your time developing and deploying websites using your platform of choice. We’re using a full-stack MEAN (MongoDB, ExpressJS, AngularJS, NodeJS) generator called generator-angular-fullstack by DaftMonk (https://github.com/DaftMonk/generator-angular-fullstack). | ||
- | ||
-Once you’ve completed the Challenge: Get Set for Back End Projects, use this guide to navigate the base structure of your new app and learn how to interact with the database as well as the user. | ||
- | ||
-[NEXT](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Guide-to-Back End Projects-Table-of-Contents) | ||
- | ||
-Sourced from (https://github.com/clnhll/guidetobasejumps) with permission. | ||
+> Hey FreeCodeCampers! This guide is here to help you navigate creating your first Back End Project. When I encountered the first Back End Project, I had no idea what was going on and spent weeks learning all of these things myself. Everything here is stuff I wish I had known coming into the Back End Projects. Oh and by the way, if you have a question that isn't answered by this guide, that's an issue, and you should report it as an issue to this repository! --[@clnhll](http://twitter.com/clnhll) | ||
+ | ||
+Yeoman is a tool that allows you to generate barebones apps based on different software stacks using "generator" packages made by developers who want to make your life easier. These packages streamline your time developing and deploying websites using your platform of choice. We're using a full-stack MEAN (MongoDB, ExpressJS, AngularJS, NodeJS) generator called generator-angular-fullstack by DaftMonk ([https://github.com/DaftMonk/generator-angular-fullstack](https://github.com/DaftMonk/generator-angular-fullstack)). | ||
+ | ||
+Once you've completed the Challenge: Get Set for Back End Projects, use this guide to navigate the base structure of your new app and learn how to interact with the database as well as the user. | ||
+ | ||
+[NEXT](https://github.com/FreeCodeCamp/FreeCodeCamp/wiki/Guide-to-Back-End-Projects-Table-of-Contents) | ||
+ | ||
+Sourced from ([https://github.com/clnhll/guidetobasejumps](https://github.com/clnhll/guidetobasejumps)) with permission. |
482
Map.md
4
Start-Here.md
175
Using-Github-Pages-for-your-front-end-development-projects.md
@@ -1,94 +1,81 @@ | ||
-@freecodecamp [asked me nicely](https://twitter.com/FreeCodeCamp/status/648707819965837312) to explain how I use github (and github pages) instead of codepen for my front-end projects. Here's the how and why of it, starting with why. | ||
- | ||
-## Benefits | ||
- | ||
-I love Codepen.io, it's a wonderful, easy-to-use tool for simple front-end experimentation. But as the fcc projects got more complex, I realized coding locally was going to save me a bunch of headaches. My text-editor and [codekit](https://incident57.com/codekit/) combo are just waaay faster. | ||
- | ||
-* Autocomplete | ||
-* Compile everything (codepen was really dragging trying to compile Jade) | ||
-* Better auto-refresh | ||
-* Built in bower | ||
-* Git versioning | ||
-* Improved screen real-estate experience | ||
- | ||
-## Git to Github | ||
- | ||
-Since I'm already saving locally, and using git for version control, I figured might as well upload to Github. Plus, Github has a fantastic, free service for front-end projects called [Github Pages](https://pages.github.com/). Just update your repo and your changes are live. | ||
- | ||
-How it works is simple. Github checks if your repository has a branch called `gh-pages` and serves any code that's sitting in that branch. No back-end stuff here, but HTML, CSS and JS work like a charm. | ||
- | ||
-## Make it Work | ||
- | ||
-### First things first | ||
- | ||
-Let's make a new folder for your project. I'll use the [Camper News](http://freecodecamp.com/challenges/Front End Project-stylize-stories-on-camper-news) project as my example. | ||
- | ||
-Got to your work directory and make a new one. You can do this in the terminal (or not). | ||
- | ||
-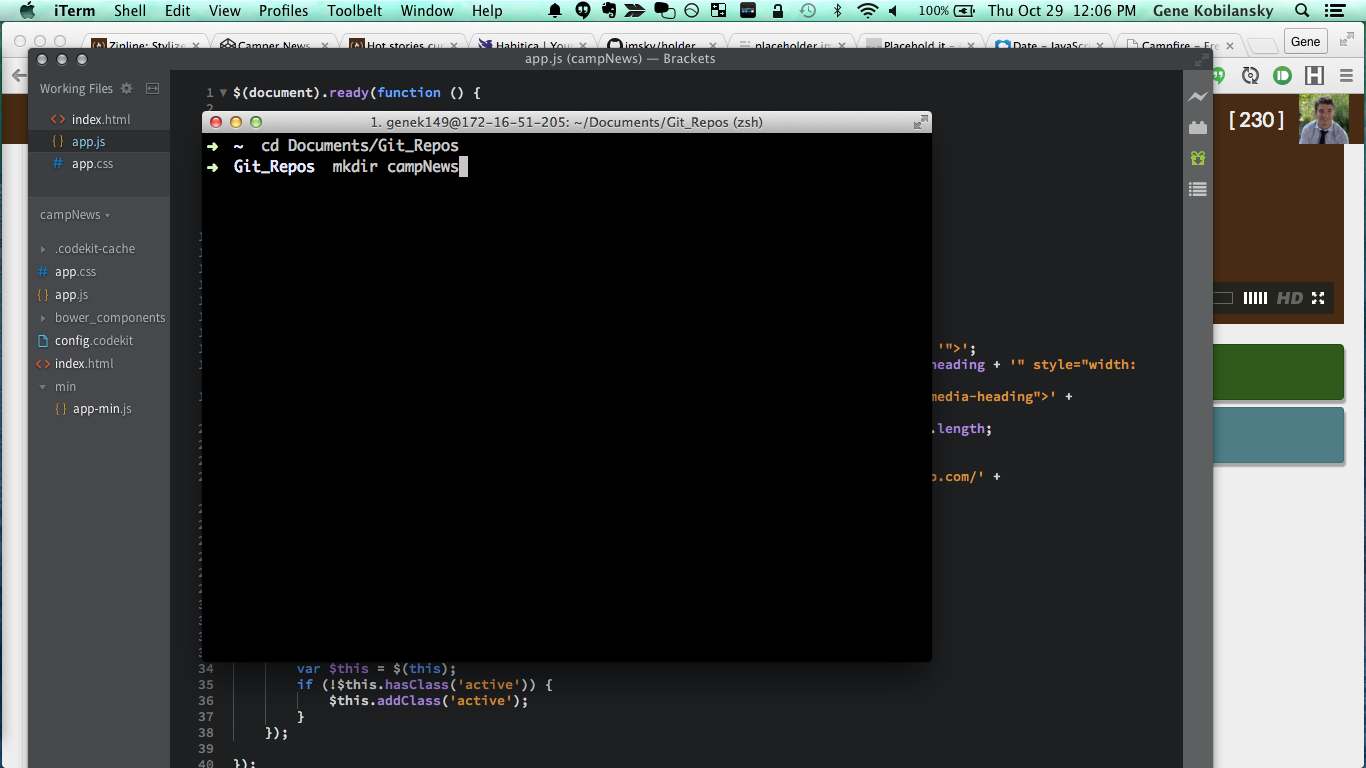 | ||
- | ||
-Now, go into the project directory, and (for sure in the terminal this time) use the command `git init`. Note, this tutorial assumes [you have git installed](https://git-scm.com/book/en/v2/Getting-Started-Installing-Git). | ||
- | ||
-Alright, awesome. Now we're ready to work. | ||
- | ||
-### Next steps | ||
- | ||
-Create some files in your campNews directory. I don't know, maybe an index.html and probably app.css and app.js, or whatever naming convention you prefer. Put your code in these files. Alright, now we're ready for our first commit. It takes **two steps**. | ||
- | ||
-1. `git add -A` will prep all these new files and the new code inside them | ||
-2. `git commit -m 'relevant message'` will commit all the work you've done to the branch you're currently on ('master' by default) | ||
- | ||
-### The key to this whole thing | ||
- | ||
-OK, so far we're still local. There's some stuff we gotta do to take our work and move it to github. It's at this point I like to switch branches. Remember - github only serves from gh-pages, and if you've followed along so far, your branch is called 'master'. Let's make a new (local) gh-pages branch. | ||
- | ||
-`git checkout -b gh-pages` will create it, copy all the work from master into gh-pages and switch me over to the branch. Phew. | ||
- | ||
-`git branch -d master` will get rid of the master branch. Sounds crazy I know, but what do we need it for? Just think of gh-pages as your NEW master branch. | ||
- | ||
-Now, `git add -A` and `git commit -m 'relevant message'` again, just in case. And be prepared to leave your editor and terminal and go online for the first time. | ||
- | ||
-Go to your github profile and create a new repo. Name it something relevant, like campNews. | ||
- | ||
-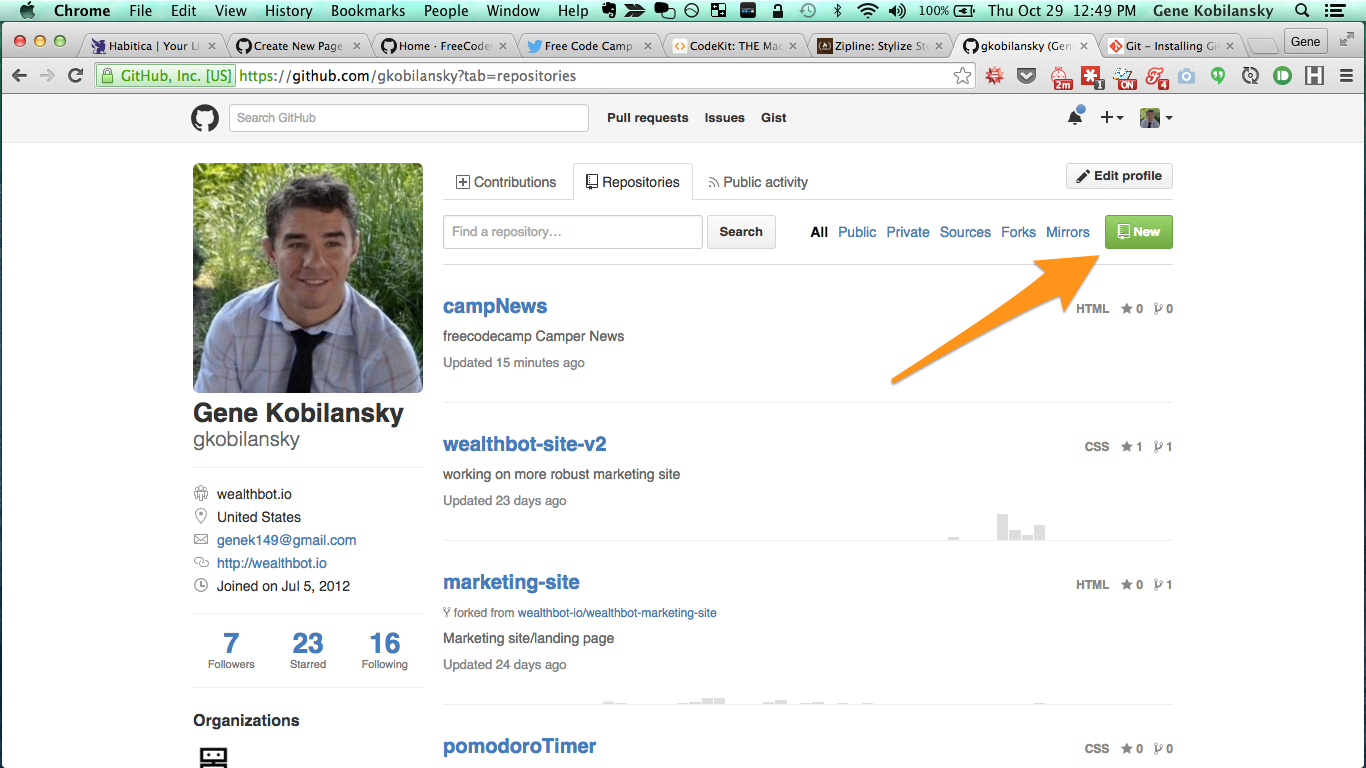 | ||
- | ||
-Once it's created, go in and grab the HTTPS clone URL. (Ignore the files in my screenshot, your repo will be empty at this point). | ||
- | ||
-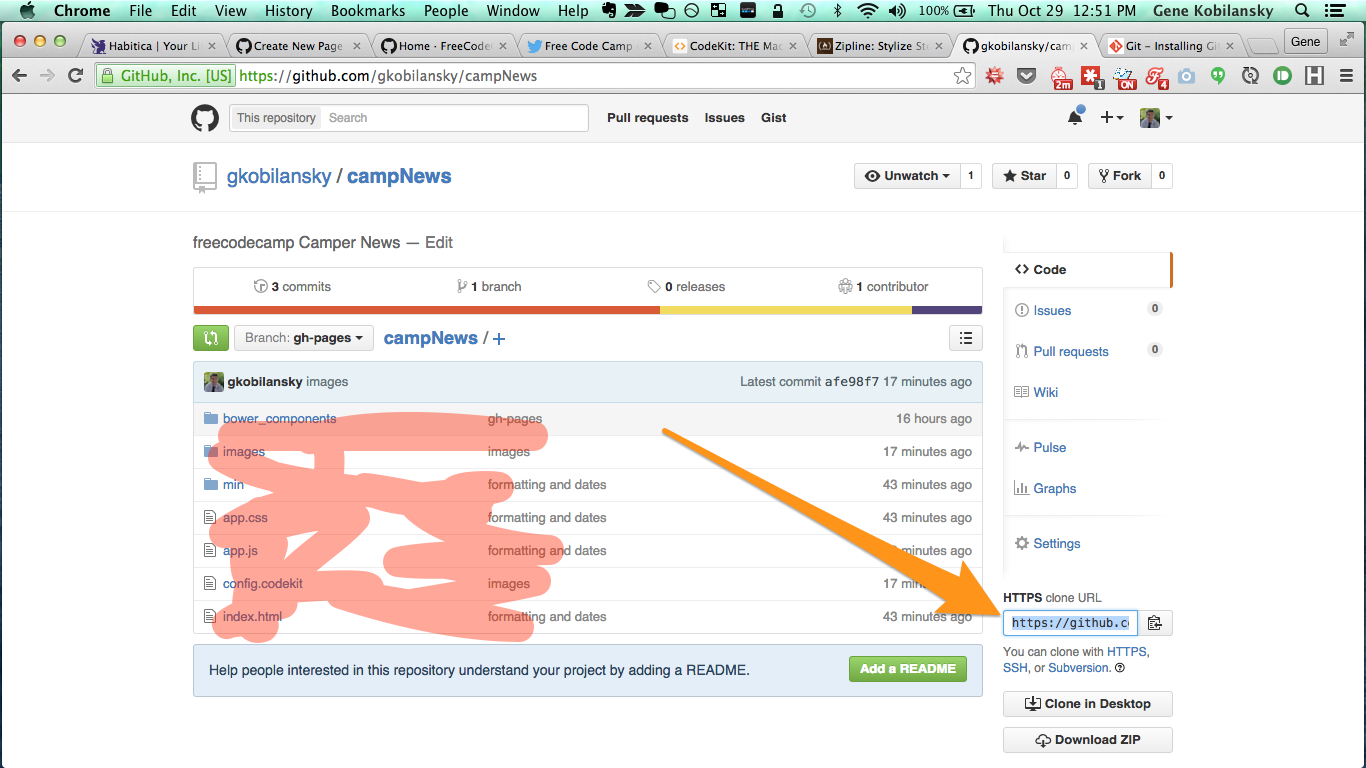 | ||
- | ||
-### Putting it all together | ||
- | ||
-And you can leave the online world. Back to the terminal! Let's connect our local project to this github repo. All it takes is one command. | ||
- | ||
-`git remote add origin <server>` Just replace server with the HTTPS url you just copied. So my command looks like this: | ||
- | ||
-`git remote add origin https://github.com/gkobilansky/campNews.git`. | ||
- | ||
-OK, so far we've: | ||
- | ||
-1. Created our project | ||
-2. Versioned it in git | ||
-3. Commited some changes | ||
-4. Switched it to the 'gh-pages' branch | ||
-5. Connected it to github | ||
- | ||
-### Last step! | ||
- | ||
-Push you project to github. Again, simple: | ||
- | ||
-`git push origin gh-pages` | ||
- | ||
-That command will make sure your latest commits get uploaded to github. Once you've done this at least once, your project should be available http://*username*.github.io/*repository*, so for me it's [http://gkobilansky.github.io/campNews](http://gkobilansky.github.io/campNews). | ||
- | ||
-Once this is all done, the process just repeats itself: | ||
- | ||
-1. `git add -A` | ||
-2. `git commit -m 'relevant message'` | ||
-3. `git push origin gh-pages` | ||
- | ||
-Granted, steeper learning curve than codepen.io, but faster and more flexible once you get the hang of it. | ||
- | ||
-Happy coding! | ||
- | ||
- | ||
-PS. Thanks to [this guide](http://rogerdudler.github.io/git-guide/) by Roger Dudler for keeping things simple. | ||
+@freecodecamp [asked me nicely](https://twitter.com/FreeCodeCamp/status/648707819965837312) to explain how I use github (and github pages) instead of codepen for my front-end projects. Here's the how and why of it, starting with why. | ||
+ | ||
+# Benefits | ||
+I love Codepen.io, it's a wonderful, easy-to-use tool for simple front-end experimentation. But as the fcc projects got more complex, I realized coding locally was going to save me a bunch of headaches. My text-editor and [codekit](https://incident57.com/codekit/) combo are just waaay faster. | ||
+- Autocomplete | ||
+- Compile everything (codepen was really dragging trying to compile Jade) | ||
+- Better auto-refresh | ||
+- Built in bower | ||
+- Git versioning | ||
+- Improved screen real-estate experience | ||
+ | ||
+# Git to Github | ||
+Since I'm already saving locally, and using git for version control, I figured might as well upload to Github. Plus, Github has a fantastic, free service for front-end projects called [Github Pages](https://pages.github.com/). Just update your repo and your changes are live. | ||
+ | ||
+How it works is simple. Github checks if your repository has a branch called `gh-pages` and serves any code that's sitting in that branch. No back-end stuff here, but HTML, CSS and JS work like a charm. | ||
+ | ||
+# Make it Work | ||
+## First things first | ||
+Let's make a new folder for your project. I'll use the [Camper News](http://www.freecodecamp.com/challenges/stylize-stories-on-camper-news) project as my example. | ||
+ | ||
+Got to your work directory and make a new one. You can do this in the terminal (or not). | ||
+ | ||
+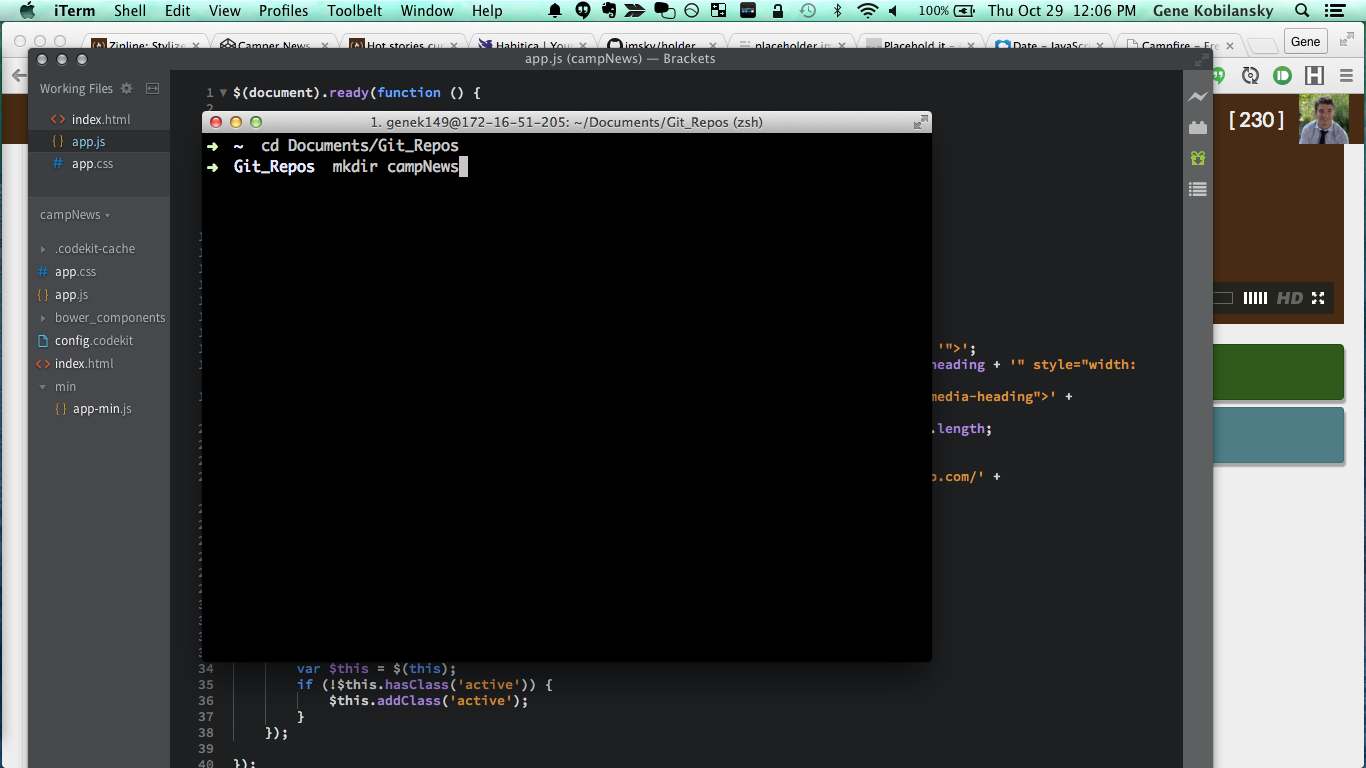 | ||
+ | ||
+Now, go into the project directory, and (for sure in the terminal this time) use the command `git init`. Note, this tutorial assumes [you have git installed](https://git-scm.com/book/en/v2/Getting-Started-Installing-Git). | ||
+ | ||
+Alright, awesome. Now we're ready to work. | ||
+ | ||
+## Next steps | ||
+Create some files in your campNews directory. I don't know, maybe an index.html and probably app.css and app.js, or whatever naming convention you prefer. Put your code in these files. Alright, now we're ready for our first commit. It takes **two steps**. | ||
+1. `git add -A` will prep all these new files and the new code inside them | ||
+2. `git commit -m 'relevant message'` will commit all the work you've done to the branch you're currently on ('master' by default) | ||
+ | ||
+## The key to this whole thing | ||
+OK, so far we're still local. There's some stuff we gotta do to take our work and move it to github. It's at this point I like to switch branches. Remember - github only serves from gh-pages, and if you've followed along so far, your branch is called 'master'. Let's make a new (local) gh-pages branch. | ||
+ | ||
+`git checkout -b gh-pages` will create it, copy all the work from master into gh-pages and switch me over to the branch. Phew. | ||
+ | ||
+`git branch -d master` will get rid of the master branch. Sounds crazy I know, but what do we need it for? Just think of gh-pages as your NEW master branch. | ||
+ | ||
+Now, `git add -A` and `git commit -m 'relevant message'` again, just in case. And be prepared to leave your editor and terminal and go online for the first time. | ||
+ | ||
+Go to your github profile and create a new repo. Name it something relevant, like campNews. | ||
+ | ||
+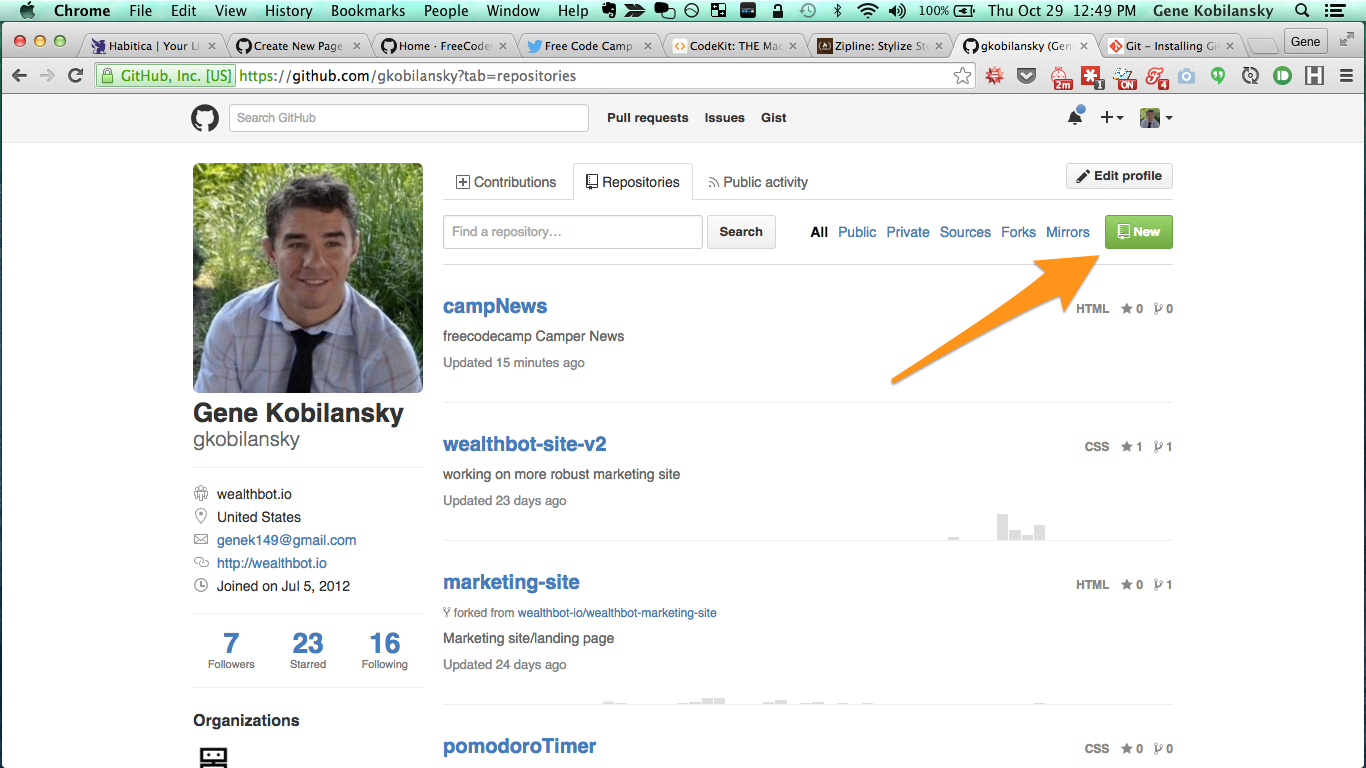 | ||
+ | ||
+Once it's created, go in and grab the HTTPS clone URL. (Ignore the files in my screenshot, your repo will be empty at this point). | ||
+ | ||
+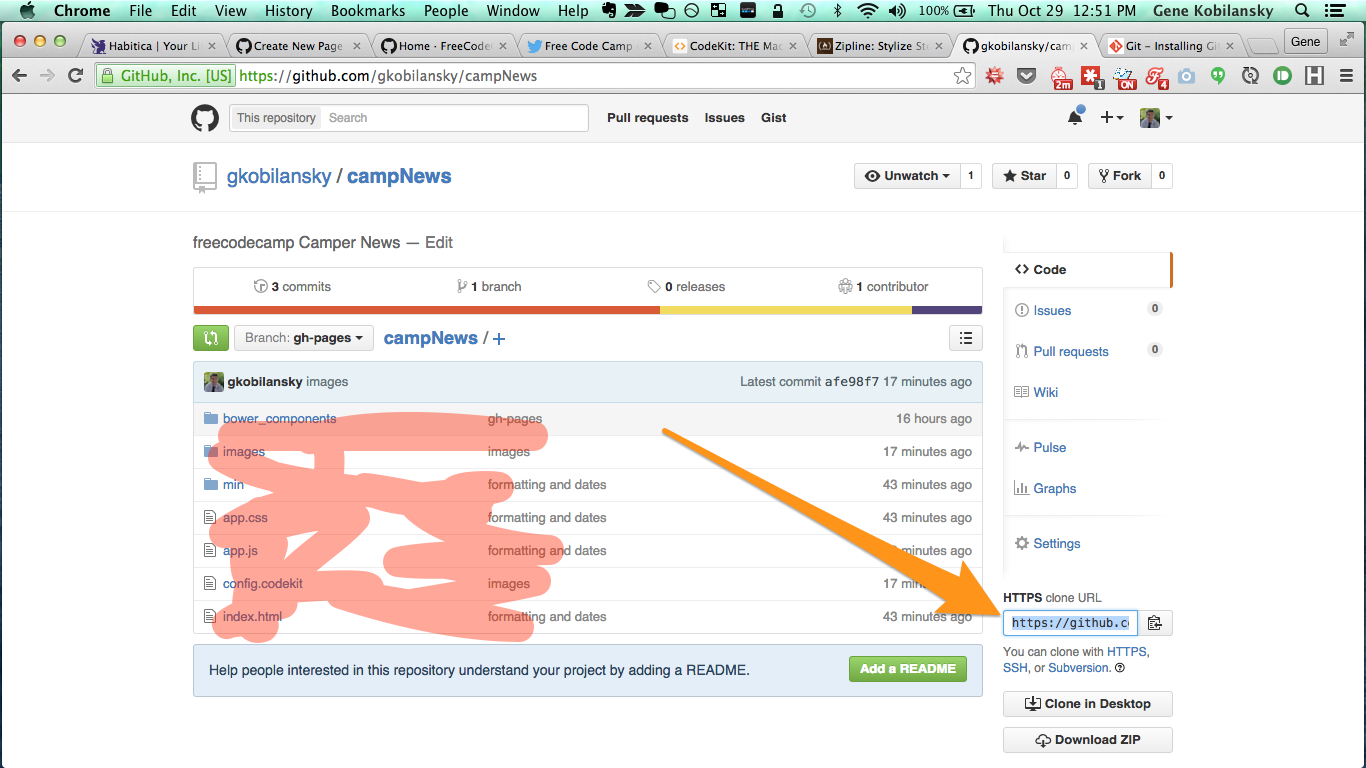 | ||
+ | ||
+## Putting it all together | ||
+And you can leave the online world. Back to the terminal! Let's connect our local project to this github repo. All it takes is one command. | ||
+ | ||
+`git remote add origin <server>` Just replace server with the HTTPS url you just copied. So my command looks like this: | ||
+ | ||
+`git remote add origin https://github.com/gkobilansky/campNews.git`. | ||
+ | ||
+OK, so far we've: | ||
+1. Created our project | ||
+2. Versioned it in git | ||
+3. Commited some changes | ||
+4. Switched it to the 'gh-pages' branch | ||
+5. Connected it to github | ||
+ | ||
+## Last step! | ||
+Push you project to github. Again, simple: | ||
+ | ||
+`git push origin gh-pages` | ||
+ | ||
+That command will make sure your latest commits get uploaded to github. Once you've done this at least once, your project should be available [http://*username*.github.io/*repository*](http://*username*.github.io/*repository*), so for me it's [http://gkobilansky.github.io/campNews](http://gkobilansky.github.io/campNews). | ||
+ | ||
+Once this is all done, the process just repeats itself: | ||
+1. `git add -A` | ||
+2. `git commit -m 'relevant message'` | ||
+3. `git push origin gh-pages` | ||
+ | ||
+Granted, steeper learning curve than codepen.io, but faster and more flexible once you get the hang of it. | ||
+ | ||
+Happy coding! | ||
+ | ||
+PS. Thanks to [this guide](http://rogerdudler.github.io/git-guide/) by Roger Dudler for keeping things simple. |
77
bf-bouncer.md
@@ -1,77 +0,0 @@ | ||
-#:fire: Algorithm: Falsy Bouncer | ||
- | ||
-# The problem | ||
- | ||
-Remove all falsy values from an array. | ||
- | ||
-Falsy values in javascript are false, null, 0, "", undefined, and NaN. | ||
- | ||
-```javascript | ||
-function bouncer(arr) { | ||
- // Don't show a false ID to this bouncer. | ||
- return arr; | ||
-} | ||
- | ||
-bouncer([7, "ate", "", false, 9]); | ||
-``` | ||
- | ||
-## Analysis | ||
-Using  to remove elements from an array. | ||
- | ||
-The _callback_ function is called for each array element to check if it will be filtered (removed). | ||
- | ||
-## Example | ||
-```javascript | ||
-function isBigEnough(value) { | ||
- return value >= 10; | ||
-} | ||
-var filtered = [12, 5, 8, 130, 44].filter(isBigEnough); | ||
-// filtered is [12, 130, 44] | ||
-``` | ||
- | ||
-## :warning: My Solution is coming up! | ||
-Don't scroll down if you don't want to see it! | ||
- | ||
- | ||
-``` | ||
- 10... | ||
- 9... | ||
- 8... | ||
- 7... | ||
- 6... | ||
- 5... | ||
- 4... | ||
- 3... | ||
- 2... | ||
-1... | ||
- | ||
-GO!!! | ||
- | ||
-``` | ||
- | ||
-## Code Solution: | ||
- | ||
-```javascript | ||
-function bouncer(arr) { | ||
- // Don't show a false ID to this bouncer. | ||
- function isNotFalsy(value) { | ||
- return value; | ||
- } | ||
- | ||
- var myArr = arr.filter(isNotFalsy); | ||
- | ||
- return myArr; | ||
-} | ||
-``` | ||
- | ||
-# References | ||
-- **Array.filter()**: [https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/filter) | ||
-- **Gorka Hernández Blog**: [https://www.gorkahernandez.com/blog/fcc-Algorithm-series-115-falsey-bouncer/](https://www.gorkahernandez.com/blog/fcc-Algorithm-series-115-falsey-bouncer/) | ||
- | ||
- | ||
-# Credits: | ||
-If you found this page useful, you can give thanks by copying and pasting this on the main chat: | ||
-`Thanks @StrongeLeeroy @HelioGuilherme66` | ||
- | ||
-> **NOTE:** Please add your username only if you have added any **relevant main contents** to the wiki page. (Please don't remove any existing usernames.) | ||
- |
6
html-elements.md
@@ -1,7 +1,7 @@ | ||
-Most HTML elements have an opening tag and a closing tag. Opening tags look like this: `<h1>`. | ||
+Most HTML elements have an opening tag and a closing tag. Opening tags look like this: `<h1>`. | ||
-Closing tags look like this: `</h1>`. | ||
+Closing tags look like this: `</h1>`. | ||
Note that the only difference between opening and closing tags is that closing tags have a slash after their opening angle bracket. | ||
-Try it here! http://www.freecodecamp.com/challenges/Challenge-say-hello-to-html-elements | ||
+Try it here! http://www.freecodecamp.com/challenges/say-hello-to-html-elements |
85
npm-debug.log
@@ -1,85 +0,0 @@ | ||
-0 info it worked if it ends with ok | ||
-1 verbose cli [ 'd:\\Program Files\\nodejs\\node.exe', | ||
-1 verbose cli 'd:\\Program Files\\nodejs\\node_modules\\npm\\bin\\npm-cli.js', | ||
-1 verbose cli 'install', | ||
-1 verbose cli 'gatsby-cli', | ||
-1 verbose cli '-g' ] | ||
-2 info using npm@2.14.7 | ||
-3 info using node@v4.2.2 | ||
-4 verbose install initial load of C:\Users\Rex\AppData\Roaming\npm\package.json | ||
-5 verbose readDependencies loading dependencies from C:\Users\Rex\AppData\Roaming\npm\package.json | ||
-6 silly cache add args [ 'gatsby-cli', null ] | ||
-7 verbose cache add spec gatsby-cli | ||
-8 silly cache add parsed spec Result { | ||
-8 silly cache add raw: 'gatsby-cli', | ||
-8 silly cache add scope: null, | ||
-8 silly cache add name: 'gatsby-cli', | ||
-8 silly cache add rawSpec: '', | ||
-8 silly cache add spec: '*', | ||
-8 silly cache add type: 'range' } | ||
-9 silly addNamed gatsby-cli@* | ||
-10 verbose addNamed "*" is a valid semver range for gatsby-cli | ||
-11 silly addNameRange { name: 'gatsby-cli', range: '*', hasData: false } | ||
-12 silly mapToRegistry name gatsby-cli | ||
-13 silly mapToRegistry using default registry | ||
-14 silly mapToRegistry registry https://registry.npmjs.org/ | ||
-15 silly mapToRegistry uri https://registry.npmjs.org/gatsby-cli | ||
-16 verbose addNameRange registry:https://registry.npmjs.org/gatsby-cli not in flight; fetching | ||
-17 verbose request uri https://registry.npmjs.org/gatsby-cli | ||
-18 verbose request no auth needed | ||
-19 info attempt registry request try #1 at 8:03:22 PM | ||
-20 verbose request id 4a2cf26426b97b9f | ||
-21 http request GET https://registry.npmjs.org/gatsby-cli | ||
-22 http 404 https://registry.npmjs.org/gatsby-cli | ||
-23 verbose headers { 'content-type': 'application/json', | ||
-23 verbose headers 'cache-control': 'max-age=0', | ||
-23 verbose headers 'content-length': '2', | ||
-23 verbose headers 'accept-ranges': 'bytes', | ||
-23 verbose headers date: 'Sun, 06 Dec 2015 04:03:24 GMT', | ||
-23 verbose headers via: '1.1 varnish', | ||
-23 verbose headers age: '0', | ||
-23 verbose headers connection: 'keep-alive', | ||
-23 verbose headers 'x-served-by': 'cache-lax1432-LAX', | ||
-23 verbose headers 'x-cache': 'MISS', | ||
-23 verbose headers 'x-cache-hits': '0', | ||
-23 verbose headers 'x-timer': 'S1449374603.909664,VS0,VE90' } | ||
-24 silly get cb [ 404, | ||
-24 silly get { 'content-type': 'application/json', | ||
-24 silly get 'cache-control': 'max-age=0', | ||
-24 silly get 'content-length': '2', | ||
-24 silly get 'accept-ranges': 'bytes', | ||
-24 silly get date: 'Sun, 06 Dec 2015 04:03:24 GMT', | ||
-24 silly get via: '1.1 varnish', | ||
-24 silly get age: '0', | ||
-24 silly get connection: 'keep-alive', | ||
-24 silly get 'x-served-by': 'cache-lax1432-LAX', | ||
-24 silly get 'x-cache': 'MISS', | ||
-24 silly get 'x-cache-hits': '0', | ||
-24 silly get 'x-timer': 'S1449374603.909664,VS0,VE90' } ] | ||
-25 verbose stack Error: Registry returned 404 for GET on https://registry.npmjs.org/gatsby-cli | ||
-25 verbose stack at makeError (d:\Program Files\nodejs\node_modules\npm\node_modules\npm-registry-client\lib\request.js:264:12) | ||
-25 verbose stack at CachingRegistryClient.<anonymous> (d:\Program Files\nodejs\node_modules\npm\node_modules\npm-registry-client\lib\request.js:242:14) | ||
-25 verbose stack at Request._callback (d:\Program Files\nodejs\node_modules\npm\node_modules\npm-registry-client\lib\request.js:172:14) | ||
-25 verbose stack at Request.self.callback (d:\Program Files\nodejs\node_modules\npm\node_modules\request\request.js:198:22) | ||
-25 verbose stack at emitTwo (events.js:87:13) | ||
-25 verbose stack at Request.emit (events.js:172:7) | ||
-25 verbose stack at Request.<anonymous> (d:\Program Files\nodejs\node_modules\npm\node_modules\request\request.js:1063:14) | ||
-25 verbose stack at emitOne (events.js:82:20) | ||
-25 verbose stack at Request.emit (events.js:169:7) | ||
-25 verbose stack at IncomingMessage.<anonymous> (d:\Program Files\nodejs\node_modules\npm\node_modules\request\request.js:1009:12) | ||
-26 verbose statusCode 404 | ||
-27 verbose pkgid gatsby-cli | ||
-28 verbose cwd d:\mean\wiki | ||
-29 error Windows_NT 10.0.10240 | ||
-30 error argv "d:\\Program Files\\nodejs\\node.exe" "d:\\Program Files\\nodejs\\node_modules\\npm\\bin\\npm-cli.js" "install" "gatsby-cli" "-g" | ||
-31 error node v4.2.2 | ||
-32 error npm v2.14.7 | ||
-33 error code E404 | ||
-34 error 404 Registry returned 404 for GET on https://registry.npmjs.org/gatsby-cli | ||
-34 error 404 | ||
-34 error 404 'gatsby-cli' is not in the npm registry. | ||
-34 error 404 You should bug the author to publish it (or use the name yourself!) | ||
-34 error 404 | ||
-34 error 404 Note that you can also install from a | ||
-34 error 404 tarball, folder, http url, or git url. | ||
-35 verbose exit [ 1, true ] |
0 comments on commit
70c868e