Get Help
Follow and Avoid Light
In this tutorial, we’ll cover how to program Sparki to follow or avoid light.You’ll Need
- A Sparki.
- A source of light (like a flashlight from the Sparki Materials Pack).
How It Works
Sparki has three light sensors that it can use to sense light: Each of Sparki’s light sensors can measure how much light is hitting them. The idea behind this follow light example is that the light is strongest on the sensor nearest to the light. Just like being further away from someone yelling makes it quieter, being further away makes the light dimmer:Strength of light is proportional to distance from the source
Programming It With Sparki

Sparki will move in the direction of the strongest light source
Following Light
Try out the example code for light following in Examples->Follow_Light menu on your SparkiDuino programming environment. This code uses basic code, integers, the light sensor, the wheel code, and if statements:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
#include <Sparki.h> // include the sparki library void setup() { } void loop() { int left = sparki.lightLeft(); // measure the left light sensor int center = sparki.lightCenter(); // measure the center light sensor int right = sparki.lightRight(); // measure the right light sensor if ( (center > left) && (center > right) ){ // if the center light is the strongest sparki.moveForward(); // move Sparki Forward } if ( (left > center) && (left > right) ){ // if the left light is the strongest sparki.moveLeft(); // move Sparki Left } if ( (right > center) && (right > left) ){ // if the right light is the strongest sparki.moveRight(); // move Sparki Right } delay(100); // wait 0.1 seconds } |
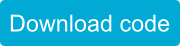
Avoiding Light
Of course, the same logic (and nearly the same program) can be used to have Sparki avoid light instead of following it: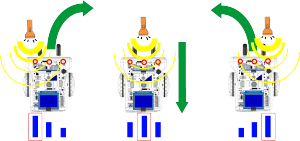
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
#include <Sparki.h> // include the sparki library void setup() { } void loop() { int left = sparki.lightLeft(); // measure the left light sensor int center = sparki.lightCenter(); // measure the center light sensor int right = sparki.lightRight(); // measure the right light sensor if ( (center > left) && (center > right) ){ // if the center light is the strongest sparki.moveBackward(); } if ( (left > center) && (left > right) ){ // if the left light is the strongest sparki.moveRight(); } if ( (right > center) && (right > left) ){ // if the right light is the strongest sparki.moveLeft(); } delay(100); // wait 0.1 seconds } |
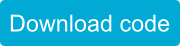
Lesson available for the miniBloq Programming Environment
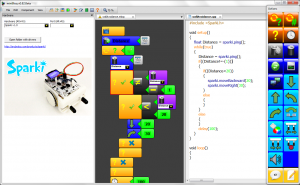